Nesnelerin, dönen üçgen gibi önceden ayarlanmış bir programa göre hareket etmesini sağlamak,
Peki, kullanıcıların OpenGL ES grafiklerinizle etkileşimde bulunmasını istiyorsanız ne yapabilirsiniz?
OpenGL ES uygulamanızı etkileşimli hale getirmenin anahtarı,
Geçersiz kılmak için GLSurfaceView
Dokunma etkinliklerini dinlemek için onTouchEvent()
.
Bu derste, kullanıcıların bir OpenGL ES nesnesini döndürmesini sağlamak için dokunma etkinliklerini nasıl dinleyeceğiniz gösterilmektedir.
Dokunmatik dinleyici kur
OpenGL ES uygulamanızın dokunma etkinliklerine yanıt vermesini sağlamak için
onTouchEvent()
yöntemi
GLSurfaceView
sınıf. Aşağıdaki örnek uygulamada, performans verilerini
MotionEvent.ACTION_MOVE
etkinlik ve bunları şu dile çevir:
şeklin dönme açısı.
Kotlin
private const val TOUCH_SCALE_FACTOR: Float = 180.0f / 320f ... private var previousX: Float = 0f private var previousY: Float = 0f override fun onTouchEvent(e: MotionEvent): Boolean { // MotionEvent reports input details from the touch screen // and other input controls. In this case, you are only // interested in events where the touch position changed. val x: Float = e.x val y: Float = e.y when (e.action) { MotionEvent.ACTION_MOVE -> { var dx: Float = x - previousX var dy: Float = y - previousY // reverse direction of rotation above the mid-line if (y > height / 2) { dx *= -1 } // reverse direction of rotation to left of the mid-line if (x < width / 2) { dy *= -1 } renderer.angle += (dx + dy) * TOUCH_SCALE_FACTOR requestRender() } } previousX = x previousY = y return true }
Java
private final float TOUCH_SCALE_FACTOR = 180.0f / 320; private float previousX; private float previousY; @Override public boolean onTouchEvent(MotionEvent e) { // MotionEvent reports input details from the touch screen // and other input controls. In this case, you are only // interested in events where the touch position changed. float x = e.getX(); float y = e.getY(); switch (e.getAction()) { case MotionEvent.ACTION_MOVE: float dx = x - previousX; float dy = y - previousY; // reverse direction of rotation above the mid-line if (y > getHeight() / 2) { dx = dx * -1 ; } // reverse direction of rotation to left of the mid-line if (x < getWidth() / 2) { dy = dy * -1 ; } renderer.setAngle( renderer.getAngle() + ((dx + dy) * TOUCH_SCALE_FACTOR)); requestRender(); } previousX = x; previousY = y; return true; }
Döndürme açısını hesapladıktan sonra bu yöntemin
Bilgilendirmek için requestRender()
oluşturucuyu değil, kareyi oluşturma zamanının geldiğini belirtir. Bu yaklaşım, bu örnekte en verimli olan
Çünkü döndürmede bir değişiklik olmadığı sürece karenin yeniden çizilmesi gerekmez. Ancak,
oluşturucudan yalnızca yeniden çizim yapmasını istemediğiniz sürece, verimlilik üzerinde herhangi bir etkisi olmaz.
Veriler, setRenderMode()
kullanılarak değişiyor.
yöntemini kullanın, dolayısıyla oluşturucuda bu satırın açıklama içermediğinden emin olun:
Kotlin
class MyGlSurfaceView(context: Context) : GLSurfaceView(context) { init { // Render the view only when there is a change in the drawing data renderMode = GLSurfaceView.RENDERMODE_WHEN_DIRTY } }
Java
public MyGLSurfaceView(Context context) { ... // Render the view only when there is a change in the drawing data setRenderMode(GLSurfaceView.RENDERMODE_WHEN_DIRTY); }
Döndürme açısını açığa çıkar
Yukarıdaki örnek kod, döndürme açısını oluşturucunuz üzerinden göstermenizi gerektirir.
herkese açık üye ekleyin. Oluşturucu kodu ana kullanıcıdan ayrı bir iş parçacığında çalıştığından
bu herkese açık değişkeni volatile
olarak bildirmeniz gerekir.
Değişkeni bildiren ve alıcı ile setter çiftini ortaya çıkaran kodu aşağıda görebilirsiniz:
Kotlin
class MyGLRenderer4 : GLSurfaceView.Renderer { @Volatile var angle: Float = 0f }
Java
public class MyGLRenderer implements GLSurfaceView.Renderer { ... public volatile float mAngle; public float getAngle() { return mAngle; } public void setAngle(float angle) { mAngle = angle; } }
Rotasyon uygula
Dokunmatik giriş tarafından oluşturulan dönüşü uygulamak için, açı oluşturan kodu yorumlayın ve Dokunmatik girişle oluşturulan açıyı içeren bir değişken ekleyin:
Kotlin
override fun onDrawFrame(gl: GL10) { ... val scratch = FloatArray(16) // Create a rotation for the triangle // long time = SystemClock.uptimeMillis() % 4000L; // float angle = 0.090f * ((int) time); Matrix.setRotateM(rotationMatrix, 0, angle, 0f, 0f, -1.0f) // Combine the rotation matrix with the projection and camera view // Note that the mvpMatrix factor *must be first* in order // for the matrix multiplication product to be correct. Matrix.multiplyMM(scratch, 0, mvpMatrix, 0, rotationMatrix, 0) // Draw triangle triangle.draw(scratch) }
Java
public void onDrawFrame(GL10 gl) { ... float[] scratch = new float[16]; // Create a rotation for the triangle // long time = SystemClock.uptimeMillis() % 4000L; // float angle = 0.090f * ((int) time); Matrix.setRotateM(rotationMatrix, 0, mAngle, 0, 0, -1.0f); // Combine the rotation matrix with the projection and camera view // Note that the vPMatrix factor *must be first* in order // for the matrix multiplication product to be correct. Matrix.multiplyMM(scratch, 0, vPMatrix, 0, rotationMatrix, 0); // Draw triangle mTriangle.draw(scratch); }
Yukarıda açıklanan adımları tamamladıktan sonra programı çalıştırın ve parmağınızı ekranın üçgeni döndürmek için:
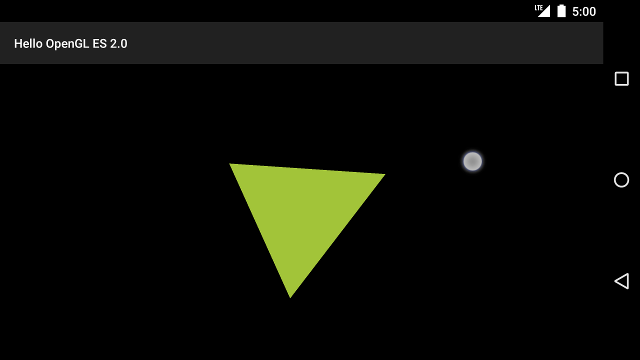
Şekil 1. Dokunmatik girişle döndürülen üçgen (daire, dokunmayı gösterir yer).