通过应用栏,您可以为用户操作添加按钮。借助此功能,您可以将当前上下文最重要的操作放在应用顶部。例如,当用户查看自己的相册时,照片浏览应用可能会在顶部显示“分享”和“创建影集”按钮。当用户查看单张照片时,应用可能会显示剪裁和滤镜按钮。
应用栏中的空间有限。如果应用声明的操作数量过多,导致应用栏无法容纳,应用栏会将多余的操作发送到溢出菜单。应用还可以指定某项操作始终显示在溢出菜单中,而不是显示在应用栏中。
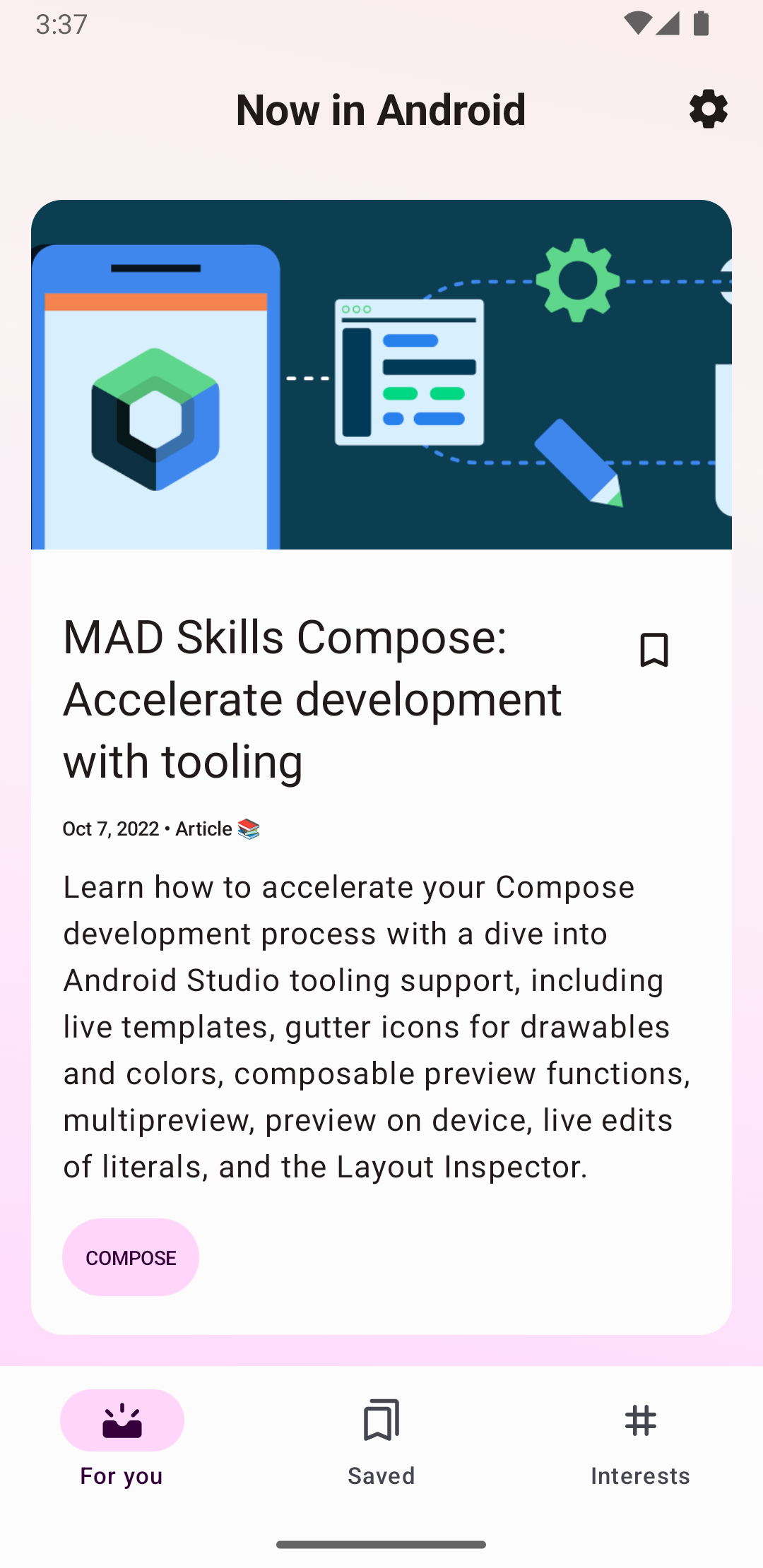
添加操作按钮
操作溢出菜单中提供的所有操作按钮和其他项均在 XML 菜单资源中定义。如需向操作栏添加操作,请在项目的 res/menu/
目录中创建一个新的 XML 文件。
为要包含在操作栏中的每一项添加一个 <item>
元素,如以下示例菜单 XML 文件中所示:
<menu xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto"> <!-- "Mark Favorite", must appear as action button if possible. --> <item android:id="@+id/action_favorite" android:icon="@drawable/ic_favorite_black_48dp" android:title="@string/action_favorite" app:showAsAction="ifRoom"/> <!-- Settings, must always be in the overflow. --> <item android:id="@+id/action_settings" android:title="@string/action_settings" app:showAsAction="never"/> </menu>
app:showAsAction
属性指定操作是否在应用栏中显示为按钮。如果您设置了 app:showAsAction="ifRoom"
(如示例代码的“收藏”操作所示),而且应用栏中有足够的空间,该操作会显示为按钮。如果没有足够的空间,系统会将超出上限的操作发送到溢出菜单。如果您设置了 app:showAsAction="never"
(如示例代码的“设置”操作所示),该操作会始终列在溢出菜单中,而不会显示在应用栏中。
如果操作显示在应用栏中,系统会将操作的图标用作操作按钮。您可以在 Material 图标中找到许多有用的图标。
响应操作
当用户选择应用栏中的某个项时,系统会调用您的 activity 的 onOptionsItemSelected()
回调方法,并传递 MenuItem
对象以指示所点按的项。在您的 onOptionsItemSelected()
实现中,调用 MenuItem.getItemId()
方法可确定点按的是哪项内容。返回的 ID 与您在相应 <item>
元素的 android:id
属性中声明的值一致。
例如,以下代码段会检查用户选择的是哪项操作。如果方法无法识别用户的操作,则会调用父类方法:
Kotlin
override fun onOptionsItemSelected(item: MenuItem) = when (item.itemId) { R.id.action_settings -> { // User chooses the "Settings" item. Show the app settings UI. true } R.id.action_favorite -> { // User chooses the "Favorite" action. Mark the current item as a // favorite. true } else -> { // The user's action isn't recognized. // Invoke the superclass to handle it. super.onOptionsItemSelected(item) } }
Java
@Override public boolean onOptionsItemSelected(MenuItem item) { switch (item.getItemId()) { case R.id.action_settings: // User chooses the "Settings" item. Show the app settings UI. return true; case R.id.action_favorite: // User chooses the "Favorite" action. Mark the current item as a // favorite. return true; default: // The user's action isn't recognized. // Invoke the superclass to handle it. return super.onOptionsItemSelected(item); } }