使用 WindowInsetsCompat
,您的应用可以查询和控制屏幕键盘(也称为 IME),就像与系统栏互动一样。您的应用还可以使用 WindowInsetsAnimationCompat
在软件键盘打开或关闭时创建流畅的转换效果。
前提条件
在为软件键盘设置控件和动画之前,请将应用配置为全屏显示。这样,它就可以处理系统窗口内边距,例如系统栏和屏幕键盘。
检查键盘软件的可见性
使用 WindowInsets
检查软件键盘的可见性。
Kotlin
val insets = ViewCompat.getRootWindowInsets(view) ?: return val imeVisible = insets.isVisible(WindowInsetsCompat.Type.ime()) val imeHeight = insets.getInsets(WindowInsetsCompat.Type.ime()).bottom
Java
WindowInsetsCompat insets = ViewCompat.getRootWindowInsets(view); boolean imeVisible = insets.isVisible(WindowInsetsCompat.Type.ime()); int imeHeight = insets.getInsets(WindowInsetsCompat.Type.ime()).bottom;
或者,您也可以使用 ViewCompat.setOnApplyWindowInsetsListener
来观察软件键盘可见性的变化。
Kotlin
ViewCompat.setOnApplyWindowInsetsListener(view) { _, insets -> val imeVisible = insets.isVisible(WindowInsetsCompat.Type.ime()) val imeHeight = insets.getInsets(WindowInsetsCompat.Type.ime()).bottom insets }
Java
ViewCompat.setOnApplyWindowInsetsListener(view, (v, insets) -> { boolean imeVisible = insets.isVisible(WindowInsetsCompat.Type.ime()); int imeHeight = insets.getInsets(WindowInsetsCompat.Type.ime()).bottom; return insets; });
将动画与软件键盘同步
当用户点按文本输入字段时,键盘会从屏幕底部滑动到位,如以下示例所示:
图 2 中标记为“未同步”的示例显示了 Android 10(API 级别 29)中的默认行为,其中应用的文本字段和内容会立即弹出到位,而不是与键盘的动画同步,这种行为在视觉上可能会令人不适。
在 Android 11(API 级别 30)及更高版本中,您可以使用
WindowInsetsAnimationCompat
将应用的转换与从屏幕底部滑动向上和向下显示的键盘同步。这样看起来会更流畅,如图 2 中标记为“同步”的示例所示。
使用要与键盘动画同步的视图配置 WindowInsetsAnimationCompat.Callback
。
Kotlin
ViewCompat.setWindowInsetsAnimationCallback( view, object : WindowInsetsAnimationCompat.Callback(DISPATCH_MODE_STOP) { // Override methods. } )
Java
ViewCompat.setWindowInsetsAnimationCallback( view, new WindowInsetsAnimationCompat.Callback( WindowInsetsAnimationCompat.Callback.DISPATCH_MODE_STOP ) { // Override methods. });
在 WindowInsetsAnimationCompat.Callback
中可以替换多种方法,即 onPrepare()
、onStart()
、onProgress()
和 onEnd()
。首先,在任何布局更改之前调用 onPrepare()
。
在开始内嵌动画时,系统会调用 onPrepare
,并在视图因动画而重新布局之前调用。您可以使用它保存开始状态,在本例中,就是视图的底部坐标。
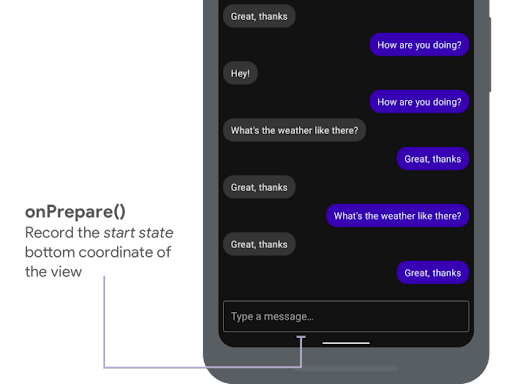
onPrepare()
记录开始状态。
以下代码段显示了 onPrepare
调用示例:
Kotlin
var startBottom = 0f override fun onPrepare( animation: WindowInsetsAnimationCompat ) { startBottom = view.bottom.toFloat() }
Java
float startBottom; @Override public void onPrepare( @NonNull WindowInsetsAnimationCompat animation ) { startBottom = view.getBottom(); }
当内嵌动画开始时,系统会调用 onStart
。您可以使用它将所有视图属性设置为布局更改的最终状态。如果您已将 OnApplyWindowInsetsListener
回调设置为任何视图,则此时系统已调用该回调。此时非常适合保存视图属性的结束状态。
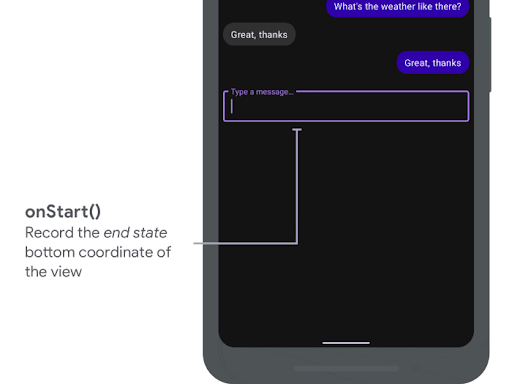
onStart()
记录结束状态。
以下代码段显示了 onStart
调用示例:
Kotlin
var endBottom = 0f override fun onStart( animation: WindowInsetsAnimationCompat, bounds: WindowInsetsAnimationCompat.BoundsCompat ): WindowInsetsAnimationCompat.BoundsCompat { // Record the position of the view after the IME transition. endBottom = view.bottom.toFloat() return bounds }
Java
float endBottom; @NonNull @Override public WindowInsetsAnimationCompat.BoundsCompat onStart( @NonNull WindowInsetsAnimationCompat animation, @NonNull WindowInsetsAnimationCompat.BoundsCompat bounds ) { endBottom = view.getBottom(); return bounds; }
在运行动画的过程中,系统会调用 onProgress
,因此您可以替换它,并在键盘动画的每一帧中收到通知。更新视图属性,以便视图动画与键盘同步。
至此,所有布局更改均已完成。例如,如果您使用 View.translationY
来移位视图,则每次调用此方法时,该值都会逐渐减小,最终达到 0
以返回原始布局位置。
onProgress()
同步动画。
以下代码段显示了 onProgress
调用示例:
Kotlin
override fun onProgress( insets: WindowInsetsCompat, runningAnimations: MutableList<WindowInsetsAnimationCompat> ): WindowInsetsCompat { // Find an IME animation. val imeAnimation = runningAnimations.find { it.typeMask and WindowInsetsCompat.Type.ime() != 0 } ?: return insets // Offset the view based on the interpolated fraction of the IME animation. view.translationY = (startBottom - endBottom) * (1 - imeAnimation.interpolatedFraction) return insets }
Java
@NonNull @Override public WindowInsetsCompat onProgress( @NonNull WindowInsetsCompat insets, @NonNull List<WindowInsetsAnimationCompat> runningAnimations ) { // Find an IME animation. WindowInsetsAnimationCompat imeAnimation = null; for (WindowInsetsAnimationCompat animation : runningAnimations) { if ((animation.getTypeMask() & WindowInsetsCompat.Type.ime()) != 0) { imeAnimation = animation; break; } } if (imeAnimation != null) { // Offset the view based on the interpolated fraction of the IME animation. view.setTranslationY((startBottom - endBottom) * (1 - imeAnimation.getInterpolatedFraction())); } return insets; }
您也可以选择替换 onEnd
。系统会在动画结束后调用此方法。现在是清理所有临时更改的好时机。
其他资源
- GitHub 上的 WindowInsetsAnimation。