按钮包含文本和/或图标,可表明当用户点按它时会引发哪种操作。
您可以通过以下三种方式之一在布局中创建按钮,具体取决于按钮是仅包含文本、仅包含图标还是同时包含文本和图标:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingLeft="16dp" android:paddingRight="16dp" android:orientation="vertical" > <Button android:id="@+id/supabutton" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="I'm a button" /> <ImageButton android:layout_width="wrap_content" android:layout_height="wrap_content" android:contentDescription="A tiny Android icon" android:src="@drawable/baseline_android_24" app:tint="#ff0000" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:drawableStart="@drawable/baseline_android_24" android:drawablePadding="4dp" android:drawableTint="#ff0000" android:text="I'm a button with an icon" /> </LinearLayout>
上述代码会生成如下内容:
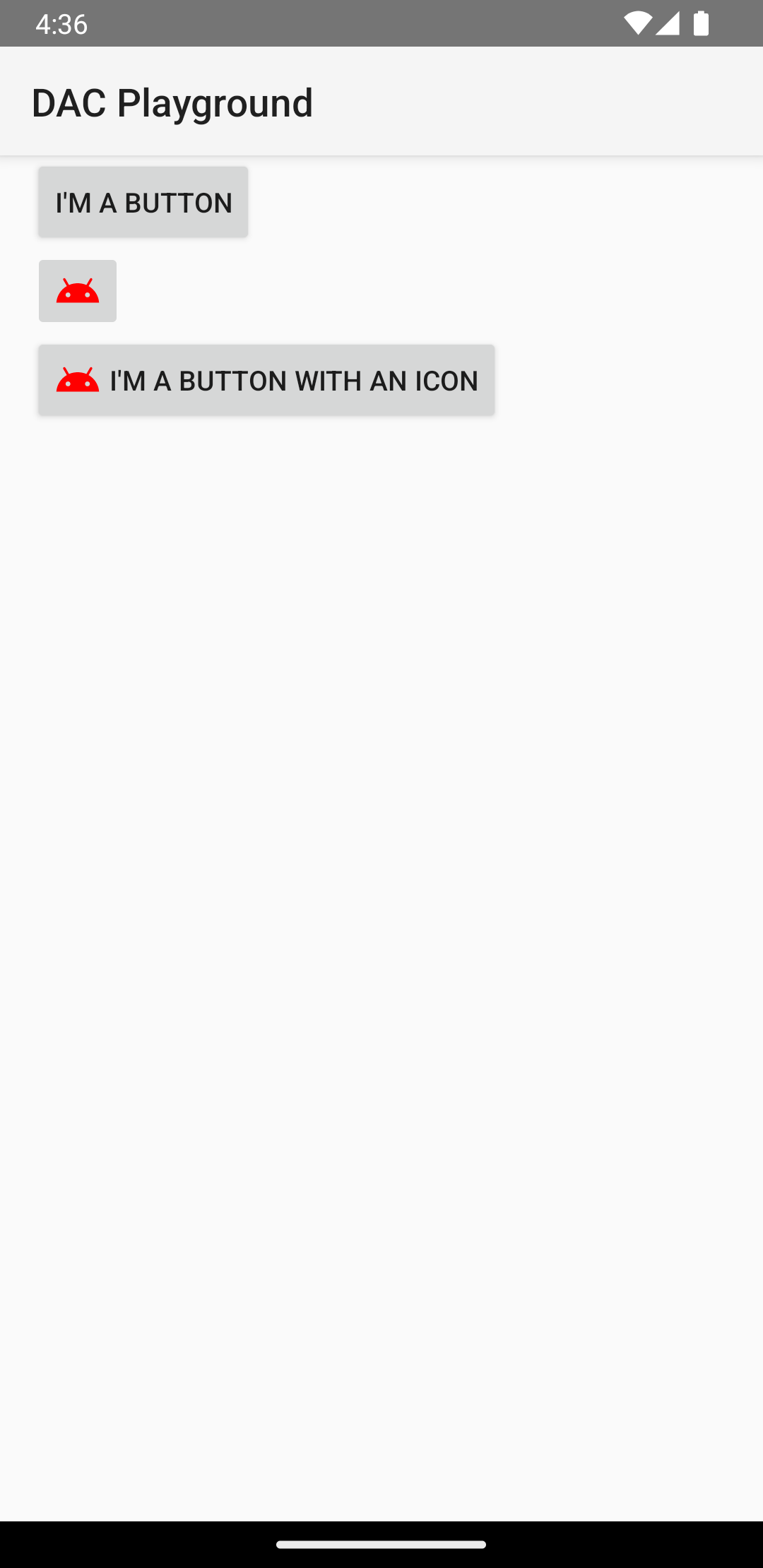
响应点击事件
当用户点按按钮时,Button
对象会收到点击事件。
如需以程序化方式声明事件处理脚本,请创建一个 View.OnClickListener
对象,并调用 setOnClickListener(View.OnClickListener)
以将其分配给按钮,如以下示例所示:
Kotlin
findViewById<Button>(R.id.supabutton) .setOnClickListener { Log.d("BUTTONS", "User tapped the Supabutton") }
Java
Button button = (Button) findViewById(R.id.supabutton); button.setOnClickListener(new View.OnClickListener() { public void onClick(View v) { Log.d("BUTTONS", "User tapped the Supabutton"); } });
设置按钮样式
按钮的外观(背景图片和字体)可能会因设备而异,因为制造商不同,设备为输入控件采用的默认样式通常也会不同。
如需使用不同背景自定义各个按钮,请使用可绘制对象或颜色资源指定 android:background
属性。或者,您可以为按钮应用样式,这样会按照与 HTML 样式类似的方式定义多个样式属性,例如背景、字体和尺寸。如需详细了解如何应用样式,请参阅样式和主题。
无边框按钮
“无边框”按钮可能会是一种非常有用的设计。无边框按钮类似于基本按钮,唯一的区别是前者没有边框或背景,但其外观仍会因状态(如点按后)而改变。
如需创建无边框按钮,请对按钮应用 borderlessButtonStyle
样式,如以下示例所示:
<Button android:id="@+id/supabutton" style="?android:attr/borderlessButtonStyle" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="I'm a button" />
自定义背景
如果要真正重新定义按钮的外观,可以指定自定义背景。不过,您的背景必须是根据按钮的当前状态更改外观的状态列表资源,而不是提供简单的位图或颜色。
您可以在 XML 文件中定义状态列表,并在其中定义三种图片或颜色,供不同的按钮状态使用。
如需为按钮背景创建状态列表可绘制资源,请执行以下操作:
- 为按钮背景创建三个位图,分别代表以下按钮状态:默认、点按和聚焦。为确保您提供的图片适合各种尺寸的按钮,请以九宫格位图形式创建位图。
- 将位图放入项目的
res/drawable/
目录中。为每个位图命名,以反映其表示的按钮状态,例如button_default.9.png
、button_pressed.9.png
和button_focused.9.png
。 - 在
res/drawable/
目录中创建一个新的 XML 文件。将其命名为button_custom.xml
之类的名称。插入如下所示的 XML:<?xml version="1.0" encoding="utf-8"?> <selector xmlns:android="http://schemas.android.com/apk/res/android"> <item android:drawable="@drawable/button_pressed" android:state_pressed="true" /> <item android:drawable="@drawable/button_focused" android:state_focused="true" /> <item android:drawable="@drawable/button_default" /> </selector>
这定义了一个可绘制资源,该资源可根据按钮的当前状态更改其图片。
- 第一个
<item>
定义点按按钮后使用的位图。 - 第二个
<item>
定义按钮处于聚焦状态时(例如使用轨迹球或方向键突出显示按钮时)使用的位图。 - 第三个
<item>
定义按钮处于默认状态(既未点按,也未聚焦)时使用的位图。
此 XML 文件表示单个可绘制资源。当
Button
引用此文件作为背景时,显示的图片将根据按钮的状态而变化。 - 第一个
- 将可绘制的 XML 文件应用为按钮背景:
<Button android:id="@+id/button_send" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/button_send" android:onClick="sendMessage" android:background="@drawable/button_custom" />
如需详细了解此 XML 语法,包括如何定义已停用、悬停或其他状态的按钮,请参阅 StateListDrawable
。