Okno dialogowe to małe okno, które zachęca użytkownika do podjęcia decyzji lub podania dodatkowych informacji. Okno dialogowe nie wypełnia ekranu i zazwyczaj służy do obsługi zdarzeń modalnych, które wymagają od użytkowników podjęcia działania, zanim będą mogli kontynuować.
Klasa Dialog
jest klasą podstawową dla dialogów, ale nie twórz instancji klasy Dialog
bezpośrednio. Zamiast tego użyj jednej z tych podklas:
AlertDialog
- Okno, które może zawierać tytuł, maksymalnie 3 przyciski, listę elementów do wyboru lub układ niestandardowy.
DatePickerDialog
lubTimePickerDialog
- Okno z wstępnie zdefiniowanym interfejsem, które umożliwia użytkownikowi wybranie daty lub godziny.
Te klasy określają styl i strukturę dialogu. Musisz też użyć
DialogFragment
jako kontenera dla dialogu. Klasa DialogFragment
udostępnia wszystkie elementy sterujące potrzebne do tworzenia okna dialogowego i zarządzania jego wyglądem zamiast wywoływania metod obiektu Dialog
.
Korzystanie z elementu DialogFragment
do zarządzania oknem dialogowym umożliwia prawidłowe obsługiwanie zdarzeń cyklu życia, np. gdy użytkownik klika przycisk Wstecz lub obraca ekran. Klasa DialogFragment
umożliwia też ponowne używanie interfejsu dialogu jako komponentu, który można osadzić w większym interfejsie (podobnie jak tradycyjny Fragment
). Może się to przydać, gdy chcesz, aby interfejs dialogu wyglądał inaczej na dużych i małych ekranach.
W poniższych sekcjach tego dokumentu opisano, jak używać obiektu DialogFragment
w połączeniu z obiektem AlertDialog
. Jeśli chcesz utworzyć selektor daty lub czasu, przeczytaj artykuł Dodawanie selektorów do aplikacji.
Tworzenie fragmentu dialogu
Możesz tworzyć różne układy dialogów, w tym niestandardowe oraz opisane w artykule Dialogi w ramach Material Design. Aby to zrobić, rozszerz DialogFragment
i utwórz funkcję AlertDialog
w metodie wywołania onCreateDialog()
.
Oto przykład podstawowej właściwości AlertDialog
zarządzanej w ramach usługi DialogFragment
:
Kotlin
class StartGameDialogFragment : DialogFragment() { override fun onCreateDialog(savedInstanceState: Bundle?): Dialog { return activity?.let { // Use the Builder class for convenient dialog construction. val builder = AlertDialog.Builder(it) builder.setMessage("Start game") .setPositiveButton("Start") { dialog, id -> // START THE GAME! } .setNegativeButton("Cancel") { dialog, id -> // User cancelled the dialog. } // Create the AlertDialog object and return it. builder.create() } ?: throw IllegalStateException("Activity cannot be null") } } class OldXmlActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_old_xml) StartGameDialogFragment().show(supportFragmentManager, "GAME_DIALOG") } }
Java
public class StartGameDialogFragment extends DialogFragment { @Override public Dialog onCreateDialog(Bundle savedInstanceState) { // Use the Builder class for convenient dialog construction. AlertDialog.Builder builder = new AlertDialog.Builder(getActivity()); builder.setMessage(R.string.dialog_start_game) .setPositiveButton(R.string.start, new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int id) { // START THE GAME! } }) .setNegativeButton(R.string.cancel, new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int id) { // User cancels the dialog. } }); // Create the AlertDialog object and return it. return builder.create(); } } // ... StartGameDialogFragment().show(supportFragmentManager, "GAME_DIALOG");
Gdy utworzysz instancję tej klasy i wywołasz show()
dla tego obiektu, pojawi się okno, jak pokazano na ilustracji poniżej.
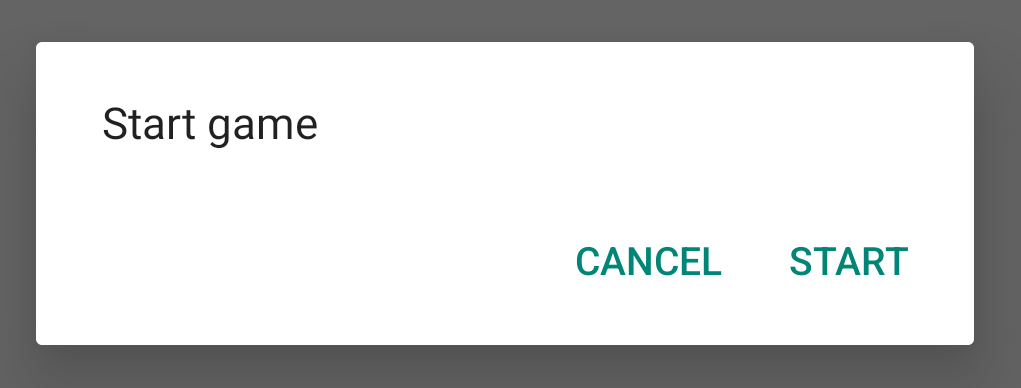
W następnej sekcji znajdziesz więcej informacji o używaniuAlertDialog.Builder
interfejsów API do tworzenia okna.
W zależności od złożoności dialogu możesz zaimplementować w DialogFragment
różne inne metody wywołania, w tym wszystkie podstawowe metody cyklu życia fragmentu.
Tworzenie okna alertu
Klasa AlertDialog
pozwala tworzyć różne projekty okien i często jest jedyną potrzebną klasą. Jak widać na rysunku poniżej, okno z ostrzeżeniem składa się z 3 obszarów:
- Tytuł: jest opcjonalny i należy go używać tylko wtedy, gdy obszar treści jest zajęty przez szczegółowy komunikat, listę lub układ niestandardowy. Jeśli chcesz przekazać prostą wiadomość lub zadać pytanie, nie musisz dodawać tytułu.
- Obszar treści: może wyświetlać wiadomość, listę lub inny niestandardowy układ.
- Przyciski działań: w dialogu może być maksymalnie 3 przyciski działań.
Klasa AlertDialog.Builder
udostępnia interfejsy API, które umożliwiają tworzenie AlertDialog
z tego typu treści, w tym z niestandardowym układem.
Aby utworzyć AlertDialog
:
Kotlin
val builder: AlertDialog.Builder = AlertDialog.Builder(context) builder .setMessage("I am the message") .setTitle("I am the title") val dialog: AlertDialog = builder.create() dialog.show()
Java
// 1. Instantiate an AlertDialog.Builder with its constructor. AlertDialog.Builder builder = new AlertDialog.Builder(getActivity()); // 2. Chain together various setter methods to set the dialog characteristics. builder.setMessage(R.string.dialog_message) .setTitle(R.string.dialog_title); // 3. Get the AlertDialog. AlertDialog dialog = builder.create();
Poprzedni fragment kodu generuje ten dialog:
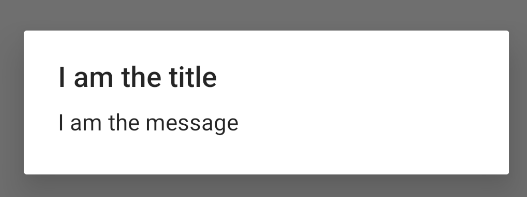
Dodawanie przycisków
Aby dodać przyciski działań takie jak na rysunku 2, wywołaj metody setPositiveButton()
i setNegativeButton()
:
Kotlin
val builder: AlertDialog.Builder = AlertDialog.Builder(context) builder .setMessage("I am the message") .setTitle("I am the title") .setPositiveButton("Positive") { dialog, which -> // Do something. } .setNegativeButton("Negative") { dialog, which -> // Do something else. } val dialog: AlertDialog = builder.create() dialog.show()
Java
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity()); // Add the buttons. builder.setPositiveButton(R.string.ok, new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int id) { // User taps OK button. } }); builder.setNegativeButton(R.string.cancel, new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int id) { // User cancels the dialog. } }); // Set other dialog properties. ... // Create the AlertDialog. AlertDialog dialog = builder.create();
Metody set...Button()
wymagają tytułu przycisku (podanego w zasobach napisów) oraz wartości DialogInterface.OnClickListener
, która określa działanie wykonywane po kliknięciu przycisku przez użytkownika.
Możesz dodać 3 przyciski poleceń:
- Pozytywne: użyj tego, aby zaakceptować i kontynuować działanie (czyli „OK”).
- Ujemna: służy do anulowania działania.
- Neutralny: użyj tego, gdy użytkownik może nie chcieć wykonać danej czynności, ale niekoniecznie chce anulować. Pojawia się między przyciskami pozytywnym i ujemnym. Może to być na przykład „Przypomnij mi później”.
Do AlertDialog
możesz dodać tylko jeden przycisk każdego typu. Na przykład nie możesz mieć więcej niż 1 przycisku „pozytywny”.
Poprzedni fragment kodu powoduje wyświetlenie okna z ostrzeżeniem, takiego jak to:
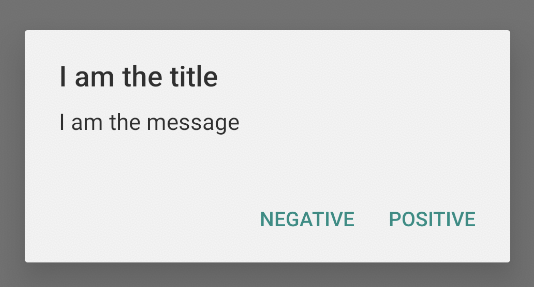
Dodaj listę
W interfejsie API AlertDialog
dostępne są 3 rodzaje list:
- tradycyjna lista jednokrotnego wyboru;
- Stała lista z jednym wyborem (przyciski).
- Stała lista wielokrotnego wyboru (pola wyboru).
Aby utworzyć listę jednokrotnego wyboru, taką jak ta na ilustracji 5, użyj metody setItems()
:
Kotlin
val builder: AlertDialog.Builder = AlertDialog.Builder(context) builder .setTitle("I am the title") .setPositiveButton("Positive") { dialog, which -> // Do something. } .setNegativeButton("Negative") { dialog, which -> // Do something else. } .setItems(arrayOf("Item One", "Item Two", "Item Three")) { dialog, which -> // Do something on item tapped. } val dialog: AlertDialog = builder.create() dialog.show()
Java
@Override public Dialog onCreateDialog(Bundle savedInstanceState) { AlertDialog.Builder builder = new AlertDialog.Builder(getActivity()); builder.setTitle(R.string.pick_color) .setItems(R.array.colors_array, new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int which) { // The 'which' argument contains the index position of the selected item. } }); return builder.create(); }
Fragment kodu generuje następujące okno dialogowe:
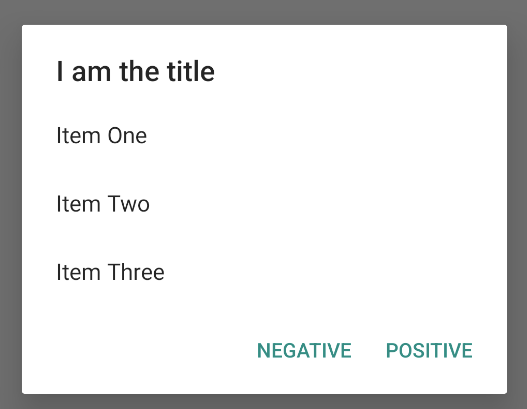
Lista jest widoczna w obszarze treści okna, więc okno nie może zawierać zarówno wiadomości, jak i listy. Ustaw tytuł okna dialogowego za pomocą setTitle()
.
Aby określić elementy listy, wywołaj funkcję setItems()
, podając tablicę. Możesz też określić listę za pomocą atrybutu setAdapter()
.
Dzięki temu możesz uzupełnić listę danymi dynamicznymi, np. z bazy danych, za pomocą funkcji ListAdapter
.
Jeśli listę zawierającą ListAdapter
, zawsze używaj Loader
, aby treści wczytywały się asynchronicznie. Więcej informacji znajdziesz w artykule Tworzenie układów za pomocą adaptera i artykule Ładowarki.
Dodaj listę trwałej lub jednokrotnego wyboru
Aby dodać listę elementów z wielokrotnie wybranymi odpowiedziami (pola wyboru) lub z pojedynczo wybranymi odpowiedziami (przyciski opcji), użyj odpowiednio metody setMultiChoiceItems()
lub setSingleChoiceItems()
.
Oto przykład utworzenia listy z kilkoma opcjami, takiej jak ta na rysunku 6, która zapisuje wybrane elementy w elementach ArrayList
:
Kotlin
val builder: AlertDialog.Builder = AlertDialog.Builder(context) builder .setTitle("I am the title") .setPositiveButton("Positive") { dialog, which -> // Do something. } .setNegativeButton("Negative") { dialog, which -> // Do something else. } .setMultiChoiceItems( arrayOf("Item One", "Item Two", "Item Three"), null) { dialog, which, isChecked -> // Do something. } val dialog: AlertDialog = builder.create() dialog.show()
Java
@Override public Dialog onCreateDialog(Bundle savedInstanceState) { selectedItems = new ArrayList(); // Where we track the selected items AlertDialog.Builder builder = new AlertDialog.Builder(getActivity()); // Set the dialog title. builder.setTitle(R.string.pick_toppings) // Specify the list array, the items to be selected by default (null for // none), and the listener through which to receive callbacks when items // are selected. .setMultiChoiceItems(R.array.toppings, null, new DialogInterface.OnMultiChoiceClickListener() { @Override public void onClick(DialogInterface dialog, int which, boolean isChecked) { if (isChecked) { // If the user checks the item, add it to the selected // items. selectedItems.add(which); } else if (selectedItems.contains(which)) { // If the item is already in the array, remove it. selectedItems.remove(which); } } }) // Set the action buttons .setPositiveButton(R.string.ok, new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialog, int id) { // User taps OK, so save the selectedItems results // somewhere or return them to the component that opens the // dialog. ... } }) .setNegativeButton(R.string.cancel, new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialog, int id) { ... } }); return builder.create(); }
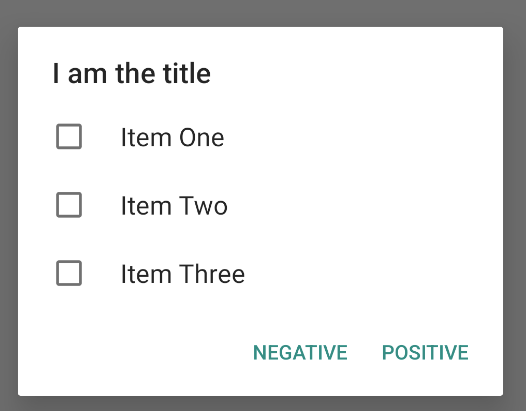
Okno alertu z jednym wyborem można uzyskać w ten sposób:
Kotlin
val builder: AlertDialog.Builder = AlertDialog.Builder(context) builder .setTitle("I am the title") .setPositiveButton("Positive") { dialog, which -> // Do something. } .setNegativeButton("Negative") { dialog, which -> // Do something else. } .setSingleChoiceItems( arrayOf("Item One", "Item Two", "Item Three"), 0 ) { dialog, which -> // Do something. } val dialog: AlertDialog = builder.create() dialog.show()
Java
String[] choices = {"Item One", "Item Two", "Item Three"}; AlertDialog.Builder builder = AlertDialog.Builder(context); builder .setTitle("I am the title") .setPositiveButton("Positive", (dialog, which) -> { }) .setNegativeButton("Negative", (dialog, which) -> { }) .setSingleChoiceItems(choices, 0, (dialog, which) -> { }); AlertDialog dialog = builder.create(); dialog.show();
W efekcie powstaje taki przykład:
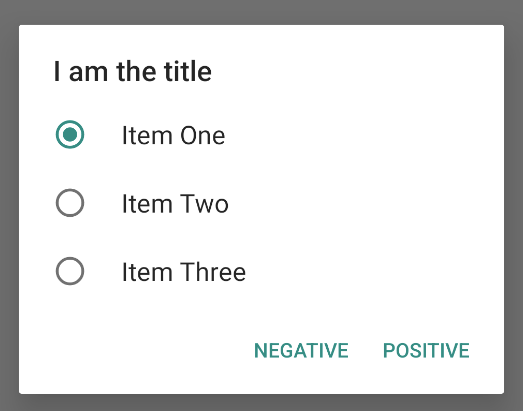
Tworzenie układu niestandardowego
Jeśli w oknie chcesz zastosować układ niestandardowy, utwórz układ i dodaj go do obiektu AlertDialog
, wywołując obiekt setView()
w obiekcie AlertDialog.Builder
.
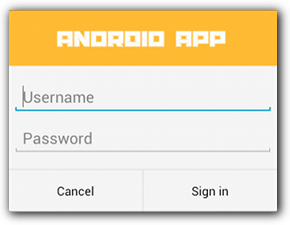
Domyślnie układ niestandardowy wypełnia okno dialogowe, ale nadal możesz używać metod AlertDialog.Builder
, aby dodawać przyciski i tytuły.
Oto na przykład plik układu dla poprzedniego niestandardowego układu okna dialogowego:
res/layout/dialog_signin.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="wrap_content" android:layout_height="wrap_content"> <ImageView android:src="@drawable/header_logo" android:layout_width="match_parent" android:layout_height="64dp" android:scaleType="center" android:background="#FFFFBB33" android:contentDescription="@string/app_name" /> <EditText android:id="@+id/username" android:inputType="textEmailAddress" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="16dp" android:layout_marginLeft="4dp" android:layout_marginRight="4dp" android:layout_marginBottom="4dp" android:hint="@string/username" /> <EditText android:id="@+id/password" android:inputType="textPassword" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="4dp" android:layout_marginLeft="4dp" android:layout_marginRight="4dp" android:layout_marginBottom="16dp" android:fontFamily="sans-serif" android:hint="@string/password"/> </LinearLayout>
Aby napompować układ w DialogFragment
, pobierz LayoutInflater
z getLayoutInflater()
i wywołaj funkcję inflate()
.
Pierwszym z nich jest identyfikator zasobu układu, a drugi to widok nadrzędny układu. Następnie możesz wywołać funkcję setView()
, aby umieścić układ w dialogu. Widać to w przykładzie poniżej.
Kotlin
override fun onCreateDialog(savedInstanceState: Bundle?): Dialog { return activity?.let { val builder = AlertDialog.Builder(it) // Get the layout inflater. val inflater = requireActivity().layoutInflater; // Inflate and set the layout for the dialog. // Pass null as the parent view because it's going in the dialog // layout. builder.setView(inflater.inflate(R.layout.dialog_signin, null)) // Add action buttons. .setPositiveButton(R.string.signin, DialogInterface.OnClickListener { dialog, id -> // Sign in the user. }) .setNegativeButton(R.string.cancel, DialogInterface.OnClickListener { dialog, id -> getDialog().cancel() }) builder.create() } ?: throw IllegalStateException("Activity cannot be null") }
Java
@Override public Dialog onCreateDialog(Bundle savedInstanceState) { AlertDialog.Builder builder = new AlertDialog.Builder(getActivity()); // Get the layout inflater. LayoutInflater inflater = requireActivity().getLayoutInflater(); // Inflate and set the layout for the dialog. // Pass null as the parent view because it's going in the dialog layout. builder.setView(inflater.inflate(R.layout.dialog_signin, null)) // Add action buttons .setPositiveButton(R.string.signin, new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialog, int id) { // Sign in the user. } }) .setNegativeButton(R.string.cancel, new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int id) { LoginDialogFragment.this.getDialog().cancel(); } }); return builder.create(); }
Jeśli chcesz wyświetlić niestandardowe okno dialogowe, możesz zamiast interfejsów API Dialog
użyć interfejsu Activity
. Utwórz aktywność i ustaw jej motyw na Theme.Holo.Dialog
w elemencie manifestu <activity>
:
<activity android:theme="@android:style/Theme.Holo.Dialog" >
Aktywność wyświetla się teraz w oknie dialogowym, a nie na pełnym ekranie.
Przekazywanie zdarzeń do gospodarza okna
Gdy użytkownik kliknie jeden z przycisków akcji w oknie lub wybierze element na liście, DialogFragment
może wykonać niezbędne działanie samodzielnie, ale często zdarza się, że zdarzenie jest przekazywane do aktywności lub fragmentu, który otwiera okno. Aby to zrobić, zdefiniuj interfejs z metodą dla każdego typu zdarzenia kliknięcia. Następnie wdróż ten interfejs w komponencie hosta, który odbiera zdarzenia działania z okna.
Oto przykładowy komponent DialogFragment
definiujący interfejs, który przekazuje zdarzenia z powrotem do działania hosta:
Kotlin
class NoticeDialogFragment : DialogFragment() { // Use this instance of the interface to deliver action events. internal lateinit var listener: NoticeDialogListener // The activity that creates an instance of this dialog fragment must // implement this interface to receive event callbacks. Each method passes // the DialogFragment in case the host needs to query it. interface NoticeDialogListener { fun onDialogPositiveClick(dialog: DialogFragment) fun onDialogNegativeClick(dialog: DialogFragment) } // Override the Fragment.onAttach() method to instantiate the // NoticeDialogListener. override fun onAttach(context: Context) { super.onAttach(context) // Verify that the host activity implements the callback interface. try { // Instantiate the NoticeDialogListener so you can send events to // the host. listener = context as NoticeDialogListener } catch (e: ClassCastException) { // The activity doesn't implement the interface. Throw exception. throw ClassCastException((context.toString() + " must implement NoticeDialogListener")) } } }
Java
public class NoticeDialogFragment extends DialogFragment { // The activity that creates an instance of this dialog fragment must // implement this interface to receive event callbacks. Each method passes // the DialogFragment in case the host needs to query it. public interface NoticeDialogListener { public void onDialogPositiveClick(DialogFragment dialog); public void onDialogNegativeClick(DialogFragment dialog); } // Use this instance of the interface to deliver action events. NoticeDialogListener listener; // Override the Fragment.onAttach() method to instantiate the // NoticeDialogListener. @Override public void onAttach(Context context) { super.onAttach(context); // Verify that the host activity implements the callback interface. try { // Instantiate the NoticeDialogListener so you can send events to // the host. listener = (NoticeDialogListener) context; } catch (ClassCastException e) { // The activity doesn't implement the interface. Throw exception. throw new ClassCastException(activity.toString() + " must implement NoticeDialogListener"); } } ... }
Aktywność zawierająca okno dialogu tworzy instancję okna dialogu za pomocą konstruktora fragmentu dialogu i odbiera zdarzenia okna dialogu za pomocą implementacji interfejsu NoticeDialogListener
:
Kotlin
class MainActivity : FragmentActivity(), NoticeDialogFragment.NoticeDialogListener { fun showNoticeDialog() { // Create an instance of the dialog fragment and show it. val dialog = NoticeDialogFragment() dialog.show(supportFragmentManager, "NoticeDialogFragment") } // The dialog fragment receives a reference to this Activity through the // Fragment.onAttach() callback, which it uses to call the following // methods defined by the NoticeDialogFragment.NoticeDialogListener // interface. override fun onDialogPositiveClick(dialog: DialogFragment) { // User taps the dialog's positive button. } override fun onDialogNegativeClick(dialog: DialogFragment) { // User taps the dialog's negative button. } }
Java
public class MainActivity extends FragmentActivity implements NoticeDialogFragment.NoticeDialogListener{ ... public void showNoticeDialog() { // Create an instance of the dialog fragment and show it. DialogFragment dialog = new NoticeDialogFragment(); dialog.show(getSupportFragmentManager(), "NoticeDialogFragment"); } // The dialog fragment receives a reference to this Activity through the // Fragment.onAttach() callback, which it uses to call the following // methods defined by the NoticeDialogFragment.NoticeDialogListener // interface. @Override public void onDialogPositiveClick(DialogFragment dialog) { // User taps the dialog's positive button. ... } @Override public void onDialogNegativeClick(DialogFragment dialog) { // User taps the dialog's negative button. ... } }
Aktywność hosta implementuje interfejsNoticeDialogListener
, który jest wymagany przez metodę wywołaniaonAttach()
pokazaną w poprzednim przykładzie. Fragment dialogu może używać metod wywołania interfejsu, aby przekazywać do aktywności zdarzenia kliknięcia:
Kotlin
override fun onCreateDialog(savedInstanceState: Bundle): Dialog { return activity?.let { // Build the dialog and set up the button click handlers. val builder = AlertDialog.Builder(it) builder.setMessage(R.string.dialog_start_game) .setPositiveButton(R.string.start, DialogInterface.OnClickListener { dialog, id -> // Send the positive button event back to the // host activity. listener.onDialogPositiveClick(this) }) .setNegativeButton(R.string.cancel, DialogInterface.OnClickListener { dialog, id -> // Send the negative button event back to the // host activity. listener.onDialogNegativeClick(this) }) builder.create() } ?: throw IllegalStateException("Activity cannot be null") }
Java
public class NoticeDialogFragment extends DialogFragment { ... @Override public Dialog onCreateDialog(Bundle savedInstanceState) { // Build the dialog and set up the button click handlers. AlertDialog.Builder builder = new AlertDialog.Builder(getActivity()); builder.setMessage(R.string.dialog_start_game) .setPositiveButton(R.string.start, new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int id) { // Send the positive button event back to the host activity. listener.onDialogPositiveClick(NoticeDialogFragment.this); } }) .setNegativeButton(R.string.cancel, new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int id) { // Send the negative button event back to the host activity. listener.onDialogNegativeClick(NoticeDialogFragment.this); } }); return builder.create(); } }
Wyświetl okno
Gdy chcesz wyświetlić dialog, utwórz instancję klasy DialogFragment
i wywołaj metodę show()
, przekazując argumenty FragmentManager
oraz nazwę tagu dla fragmentu dialogu.
Możesz uzyskać FragmentManager
, dzwoniąc pod numer
getSupportFragmentManager()
z poziomu
FragmentActivity
lub dzwoniąc pod numer
getParentFragmentManager()
z poziomu Fragment
. Oto przykład:
Kotlin
fun confirmStartGame() { val newFragment = StartGameDialogFragment() newFragment.show(supportFragmentManager, "game") }
Java
public void confirmStartGame() { DialogFragment newFragment = new StartGameDialogFragment(); newFragment.show(getSupportFragmentManager(), "game"); }
Drugi argument, "game"
, to unikalna nazwa tagu, której system używa do zapisywania i przywracania stanu fragmentu w razie potrzeby. Tag umożliwia też uzyskanie uchwytu fragmentu przez wywołanie funkcji findFragmentByTag()
.
Wyświetlanie okna dialogowego na pełnym ekranie lub jako wbudowany fragment
W niektórych sytuacjach część projektu interfejsu może wyświetlać się jako okno dialogowe, a w innych jako fragment pełnoekranowy lub osadzony. Możesz też chcieć, aby wyglądała inaczej w zależności od rozmiaru ekranu urządzenia. Klasa DialogFragment
zapewnia elastyczność w tym zakresie, ponieważ może działać jak element Fragment
, który można osadzić.
W tym przypadku nie możesz jednak tworzyć dialogów za pomocą obiektów AlertDialog.Builder
ani innych obiektów Dialog
. Jeśli chcesz, aby DialogFragment
można było osadzić, zdefiniuj interfejs użytkownika dialogu w layoutie, a następnie załaduj ten layout w wywołaniu zwrotnym onCreateView()
.
Oto przykład DialogFragment
, który może wyświetlać się jako dialog lub fragment do osadzenia, korzystając z schematu o nazwie purchase_items.xml
:
Kotlin
class CustomDialogFragment : DialogFragment() { // The system calls this to get the DialogFragment's layout, regardless of // whether it's being displayed as a dialog or an embedded fragment. override fun onCreateView( inflater: LayoutInflater, container: ViewGroup?, savedInstanceState: Bundle? ): View { // Inflate the layout to use as a dialog or embedded fragment. return inflater.inflate(R.layout.purchase_items, container, false) } // The system calls this only when creating the layout in a dialog. override fun onCreateDialog(savedInstanceState: Bundle): Dialog { // The only reason you might override this method when using // onCreateView() is to modify the dialog characteristics. For example, // the dialog includes a title by default, but your custom layout might // not need it. Here, you can remove the dialog title, but you must // call the superclass to get the Dialog. val dialog = super.onCreateDialog(savedInstanceState) dialog.requestWindowFeature(Window.FEATURE_NO_TITLE) return dialog } }
Java
public class CustomDialogFragment extends DialogFragment { // The system calls this to get the DialogFragment's layout, regardless of // whether it's being displayed as a dialog or an embedded fragment. @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { // Inflate the layout to use as a dialog or embedded fragment. return inflater.inflate(R.layout.purchase_items, container, false); } // The system calls this only when creating the layout in a dialog. @Override public Dialog onCreateDialog(Bundle savedInstanceState) { // The only reason you might override this method when using // onCreateView() is to modify the dialog characteristics. For example, // the dialog includes a title by default, but your custom layout might // not need it. Here, you can remove the dialog title, but you must // call the superclass to get the Dialog. Dialog dialog = super.onCreateDialog(savedInstanceState); dialog.requestWindowFeature(Window.FEATURE_NO_TITLE); return dialog; } }
Ten przykład określa, czy fragment ma się wyświetlać w oknie, czy w trybie pełnoekranowym, w zależności od rozmiaru ekranu:
Kotlin
fun showDialog() { val fragmentManager = supportFragmentManager val newFragment = CustomDialogFragment() if (isLargeLayout) { // The device is using a large layout, so show the fragment as a // dialog. newFragment.show(fragmentManager, "dialog") } else { // The device is smaller, so show the fragment fullscreen. val transaction = fragmentManager.beginTransaction() // For a polished look, specify a transition animation. transaction.setTransition(FragmentTransaction.TRANSIT_FRAGMENT_OPEN) // To make it fullscreen, use the 'content' root view as the container // for the fragment, which is always the root view for the activity. transaction .add(android.R.id.content, newFragment) .addToBackStack(null) .commit() } }
Java
public void showDialog() { FragmentManager fragmentManager = getSupportFragmentManager(); CustomDialogFragment newFragment = new CustomDialogFragment(); if (isLargeLayout) { // The device is using a large layout, so show the fragment as a // dialog. newFragment.show(fragmentManager, "dialog"); } else { // The device is smaller, so show the fragment fullscreen. FragmentTransaction transaction = fragmentManager.beginTransaction(); // For a polished look, specify a transition animation. transaction.setTransition(FragmentTransaction.TRANSIT_FRAGMENT_OPEN); // To make it fullscreen, use the 'content' root view as the container // for the fragment, which is always the root view for the activity. transaction.add(android.R.id.content, newFragment) .addToBackStack(null).commit(); } }
Więcej informacji o wykonaniu transakcji fragmentów znajdziesz w artykule Fragmenty.
W tym przykładzie wartość logiczna mIsLargeLayout
określa, czy bieżące urządzenie musi korzystać z dużego układu aplikacji i dlatego wyświetlać ten fragment jako okno, a nie pełny ekran. Najlepszym sposobem na ustawienie tego typu wartości logicznej jest zadeklarowanie wartości zasobu boolowskiego z wartością zasobu alternatywnego dla różnych rozmiarów ekranu. Oto 2 wersje zasobu logicznego dla różnych rozmiarów ekranu:
res/values/bools.xml
<!-- Default boolean values --> <resources> <bool name="large_layout">false</bool> </resources>
res/values-large/bools.xml
<!-- Large screen boolean values --> <resources> <bool name="large_layout">true</bool> </resources>
Następnie możesz zainicjować wartość mIsLargeLayout
w metodzie onCreate()
aktywności, jak pokazano w tym przykładzie:
Kotlin
override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) isLargeLayout = resources.getBoolean(R.bool.large_layout) }
Java
boolean isLargeLayout; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); isLargeLayout = getResources().getBoolean(R.bool.large_layout); }
Pokazuj aktywność w oknie na dużych ekranach
Zamiast wyświetlać okno jako pełnoekranowy interfejs na małych ekranach, możesz uzyskać taki sam efekt, wyświetlając Activity
w postaci okna na dużych ekranach. Wybór podejścia zależy od projektu aplikacji, ale wyświetlanie aktywności w oknie dialogowym jest często przydatne, gdy aplikacja jest przeznaczona do małych ekranów i chcesz poprawić komfort korzystania z niej na tabletach, wyświetlając krótkotrwałą aktywność w oknie dialogowym.
Aby aktywność była wyświetlana jako okno dialogowe tylko na dużych ekranach, zastosuj motyw Theme.Holo.DialogWhenLarge
do elementu <activity>
w pliku manifestu:
<activity android:theme="@android:style/Theme.Holo.DialogWhenLarge" >
Więcej informacji o stylizowaniu aktywności za pomocą motywów znajdziesz w artykule Style i motywy.
Zamykanie okna
Gdy użytkownik kliknie przycisk polecenia utworzony za pomocą AlertDialog.Builder
, system zamknie to okno za Ciebie.
System zamyka okno dialogowe, gdy użytkownik kliknie element na liście, z wyjątkiem sytuacji, gdy lista zawiera przyciski radiowe lub pola wyboru. Możesz też zamknąć to okno ręcznie, wywołując funkcję dismiss()
na urządzeniu DialogFragment
.
Jeśli musisz wykonać określone działania, gdy okno przestanie się wyświetlać, w DialogFragment
możesz wdrożyć metodę onDismiss()
.
Możesz też anulować okno. Jest to specjalne zdarzenie, które wskazuje, że użytkownik opuszcza okno, nie wykonując zadania. Dzieje się tak, gdy użytkownik kliknie przycisk Wstecz lub kliknie ekran poza obszarem okna, albo gdy jawnie wywołasz metodę cancel()
na obiekcie Dialog
, na przykład w odpowiedzi na kliknięcie przycisku „Anuluj” w oknie.
Jak widać w poprzednim przykładzie, możesz zareagować na zdarzenie anulowania, implementując onCancel()
w klasie DialogFragment
.