Android 12.0(API レベル 31)以降では、
CallStyle
通知テンプレート(着信通知と
できます。このテンプレートを使用して、VPC ファイアウォールの
進行中の着信通知。テンプレートは大きな形式の通知をサポートしている
応答や応答などの必要なアクションを含む
通話を拒否できます。
着信と通話は優先度の高いイベントであるため、これらの通知は 通知シェードで最優先されます。このランキングによって 優先通話が他のデバイスに転送されます。
CallStyle
通知テンプレートには、次の必要なアクションが含まれています。
- 着信を [応答] または [拒否] します。
- 通話中は通話を終了します。
- 電話に出るか電話を切って、通話スクリーニングを行います。
このスタイルのアクションはボタンとして表示され、システムによってアクションが自動的に 適切なアイコンとテキストが必要です。ボタンに手動でラベルを付けることはできません。 通知の設計原則について詳しくは、このモジュールの 通知。
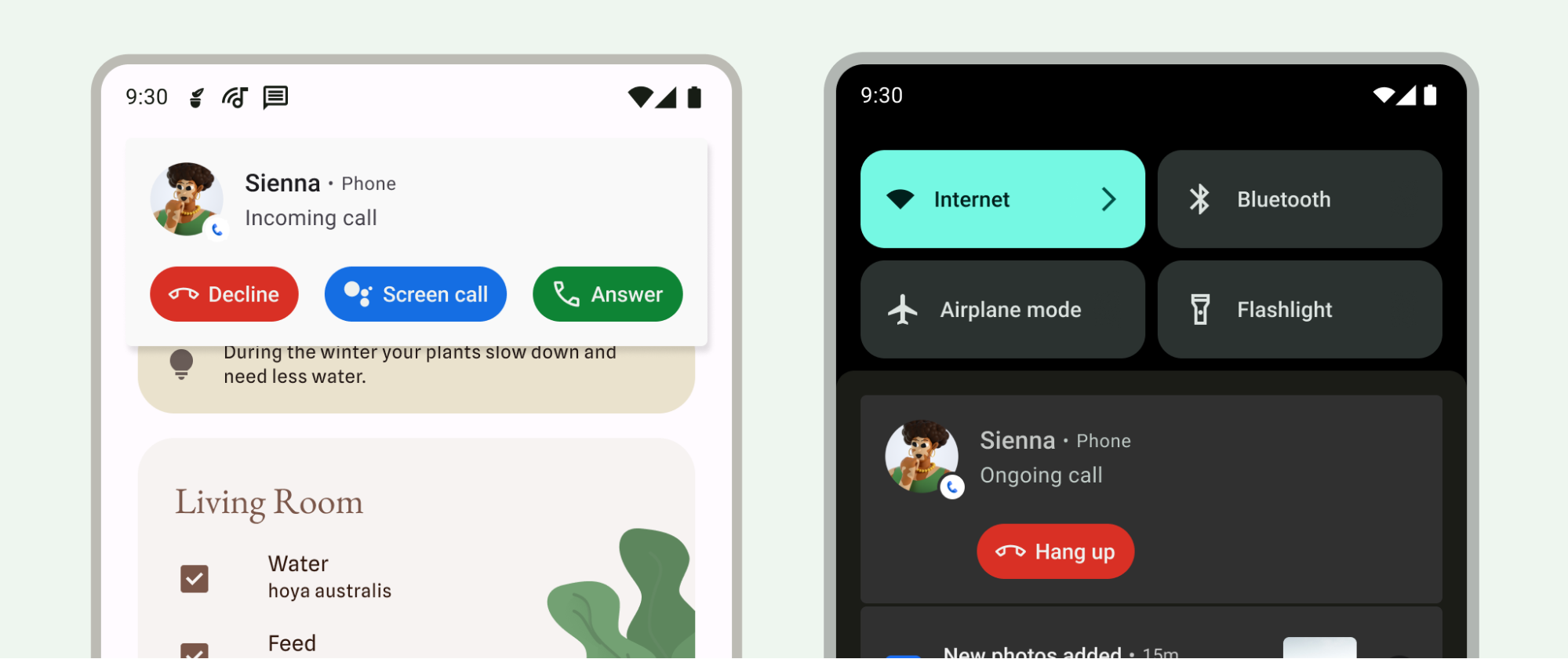
必要なアクションは、hangupIntent
や
以降のセクションで answerIntent
を使用します。これらはそれぞれが
維持します。トークンは軽量のオブジェクトで、
渡すことが可能です。このシステムは
トークンの存続期間を管理し、トークンの有効期間が
PendingIntent
は、作成元のアプリが不要になった場合でも使用可能
できます。別のアプリに PendingIntent
を割り当てると、
拒否や応答など、指定された操作を実行する権限。
この権限は、インテントを作成したアプリであっても付与されます。
は現在実行されていません。詳細については、リファレンス ドキュメントをご覧ください。
PendingIntent
。
Android 14(API レベル 34)以降では、着信通知を設定できます
閉じることができないようにします。これを行うには、CallStyle
通知を使用して、
Notification.FLAG_ONGOING_EVENT
~
Notification.Builder#setOngoing(true)
。
CallStyle
でのさまざまなメソッドの使用例を次に示します。
通知を受け取ります。
Kotlin
// Create a new call, setting the user as the caller. val incomingCaller = Person.Builder() .setName("Jane Doe") .setImportant(true) .build()
Java
// Create a new call with the user as the caller. Person incomingCaller = new Person.Builder() .setName("Jane Doe") .setImportant(true) .build();
着信
forIncomingCall()
メソッドを使用して、特定の通話タイプの通知を作成します。
着信を通知します。
Kotlin
// Create a call style notification for an incoming call. val builder = Notification.Builder(context, CHANNEL_ID) .setContentIntent(contentIntent) .setSmallIcon(smallIcon) .setStyle( Notification.CallStyle.forIncomingCall(caller, declineIntent, answerIntent)) .addPerson(incomingCaller)
Java
// Create a call style notification for an incoming call. Notification.Builder builder = Notification.Builder(context, CHANNEL_ID) .setContentIntent(contentIntent) .setSmallIcon(smallIcon) .setStyle( Notification.CallStyle.forIncomingCall(caller, declineIntent, answerIntent)) .addPerson(incomingCaller);
通話中
forOngoingCall()
メソッドを使用して、特定の通話タイプの通知を作成します。
通話中です。
Kotlin
// Create a call style notification for an ongoing call. val builder = Notification.Builder(context, CHANNEL_ID) .setContentIntent(contentIntent) .setSmallIcon(smallIcon) .setStyle( Notification.CallStyle.forOngoingCall(caller, hangupIntent)) .addPerson(second_caller)
Java
// Create a call style notification for an ongoing call. Notification.Builder builder = new Notification.Builder(context, CHANNEL_ID) .setContentIntent(contentIntent) .setSmallIcon(smallIcon) .setStyle( Notification.CallStyle.forOngoingCall(caller, hangupIntent)) .addPerson(second_caller);
通話をスクリーニングする
forScreeningCall()
メソッドを使用して、以下の通話スタイル通知を作成します。
通話をスクリーニングします。
Kotlin
// Create a call style notification for screening a call. val builder = Notification.Builder(context, CHANNEL_ID) .setContentIntent(contentIntent) .setSmallIcon(smallIcon) .setStyle( Notification.CallStyle.forScreeningCall(caller, hangupIntent, answerIntent)) .addPerson(second_caller)
Java
// Create a call style notification for screening a call. Notification.Builder builder = new Notification.Builder(context, CHANNEL_ID) .setContentIntent(contentIntent) .setSmallIcon(smallIcon) .setStyle( Notification.CallStyle.forScreeningCall(caller, hangupIntent, answerIntent)) .addPerson(second_caller);
より多くの Android バージョン間で互換性を提供する
API バージョン 30 以前の CallStyle
通知を、
API で提供された高いランクを割り当てるためにフォアグラウンド サービスを使用
レベル 31 以降である必要があります。さらに、API バージョン 30 での CallStyle
通知
以前のようにランキングを上げるには、通知を
色を付けるには setColorized()
メソッドを使用します。
CallStyle
通知で Telecom API を使用します。詳細については、次をご覧ください:
通信フレームワークの概要。