一部のコンテンツは、ステータスバーやナビゲーション バーにインジケーターを表示せずに全画面表示で視聴するのが最適です。たとえば、動画、ゲーム、画像ギャラリー、書籍、プレゼンテーションのスライドなどです。これは「没入モード」と呼ばれます。このページでは、全画面表示を利用してユーザーに没入型のコンテンツを提供し、ユーザーのエンゲージメントを高める方法について説明します。
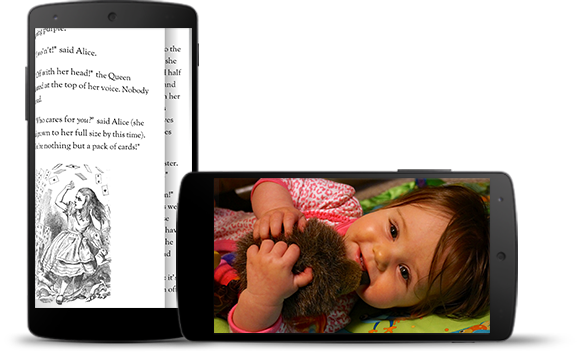
没入モードは、ゲーム中に誤って終了しないようにし、画像、動画、書籍を没入型で楽しむことができます。ただし、ユーザーが通知の確認、急な検索、その他の操作のためにアプリを出たり入ったりすること頻度に注意してください。没入モードでは、ユーザーがシステム ナビゲーションに簡単にアクセスできなくなるため、没入モードは、画面領域を広く使う以上のユーザー エクスペリエンスのメリットがある場合にのみ使用してください。
WindowInsetsControllerCompat.hide()
を使用してシステムバーを非表示にし、WindowInsetsControllerCompat.show()
を使用してシステムバーを表示します。
次のスニペットは、システムバーを非表示または表示するようにボタンを構成する例を示しています。
Kotlin
override fun onCreate(savedInstanceState: Bundle?) { ... val windowInsetsController = WindowCompat.getInsetsController(window, window.decorView) // Configure the behavior of the hidden system bars. windowInsetsController.systemBarsBehavior = WindowInsetsControllerCompat.BEHAVIOR_SHOW_TRANSIENT_BARS_BY_SWIPE // Add a listener to update the behavior of the toggle fullscreen button when // the system bars are hidden or revealed. ViewCompat.setOnApplyWindowInsetsListener(window.decorView) { view, windowInsets -> // You can hide the caption bar even when the other system bars are visible. // To account for this, explicitly check the visibility of navigationBars() // and statusBars() rather than checking the visibility of systemBars(). if (windowInsets.isVisible(WindowInsetsCompat.Type.navigationBars()) || windowInsets.isVisible(WindowInsetsCompat.Type.statusBars())) { binding.toggleFullscreenButton.setOnClickListener { // Hide both the status bar and the navigation bar. windowInsetsController.hide(WindowInsetsCompat.Type.systemBars()) } } else { binding.toggleFullscreenButton.setOnClickListener { // Show both the status bar and the navigation bar. windowInsetsController.show(WindowInsetsCompat.Type.systemBars()) } } ViewCompat.onApplyWindowInsets(view, windowInsets) } }
Java
@Override protected void onCreate(Bundle savedInstanceState) { ... WindowInsetsControllerCompat windowInsetsController = WindowCompat.getInsetsController(getWindow(), getWindow().getDecorView()); // Configure the behavior of the hidden system bars. windowInsetsController.setSystemBarsBehavior( WindowInsetsControllerCompat.BEHAVIOR_SHOW_TRANSIENT_BARS_BY_SWIPE ); // Add a listener to update the behavior of the toggle fullscreen button when // the system bars are hidden or revealed. ViewCompat.setOnApplyWindowInsetsListener( getWindow().getDecorView(), (view, windowInsets) -> { // You can hide the caption bar even when the other system bars are visible. // To account for this, explicitly check the visibility of navigationBars() // and statusBars() rather than checking the visibility of systemBars(). if (windowInsets.isVisible(WindowInsetsCompat.Type.navigationBars()) || windowInsets.isVisible(WindowInsetsCompat.Type.statusBars())) { binding.toggleFullscreenButton.setOnClickListener(v -> { // Hide both the status bar and the navigation bar. windowInsetsController.hide(WindowInsetsCompat.Type.systemBars()); }); } else { binding.toggleFullscreenButton.setOnClickListener(v -> { // Show both the status bar and the navigation bar. windowInsetsController.show(WindowInsetsCompat.Type.systemBars()); }); } return ViewCompat.onApplyWindowInsets(view, windowInsets); }); }
必要に応じて、非表示にするシステムバーのタイプを指定し、ユーザーが操作したときのバーの動作を決定できます。
非表示にするシステムバーを指定する
非表示にするシステムバーのタイプを指定するには、次のいずれかのパラメータを WindowInsetsControllerCompat.hide()
に渡します。
WindowInsetsCompat.Type.systemBars()
を使用して、両方のシステムバーを非表示にします。WindowInsetsCompat.Type.statusBars()
を使用して、ステータスバーのみを非表示にします。WindowInsetsCompat.Type.navigationBars()
を使用すると、ナビゲーション バーのみを非表示にできます。
非表示のシステムバーの動作を指定する
WindowInsetsControllerCompat.setSystemBarsBehavior()
を使用して、ユーザーが非表示のシステムバーを操作したときのバーの動作を指定します。
WindowInsetsControllerCompat.BEHAVIOR_SHOW_BARS_BY_TOUCH
を使用して、対応するディスプレイでユーザーが任意の操作を行うと、非表示のシステムバーを表示します。WindowInsetsControllerCompat.BEHAVIOR_SHOW_BARS_BY_SWIPE
を使用すると、システムバーが隠れている画面の端からスワイプするなど、任意のシステム ジェスチャーで隠れたシステムバーを表示できます。WindowInsetsControllerCompat.BEHAVIOR_SHOW_TRANSIENT_BARS_BY_SWIPE
を使用して、システム ジェスチャー(バーが隠れている画面の端からスワイプするなど)で、非表示のシステムバーを一時的に表示します。これらの一時的なシステムバーはアプリのコンテンツに重ねて表示されます。透明度を設定することもできます。また、短いタイムアウト後に自動的に非表示になります。