Per alcuni contenuti è preferibile l'esperienza a schermo intero senza indicatori nella barra di stato o nella barra di navigazione. Alcuni esempi sono video, giochi, gallerie di immagini, libri e diapositive di presentazioni. Questa modalità è nota come modalità immersiva. Questa pagina mostra come coinvolgere più a fondo gli utenti con i contenuti a schermo intero.
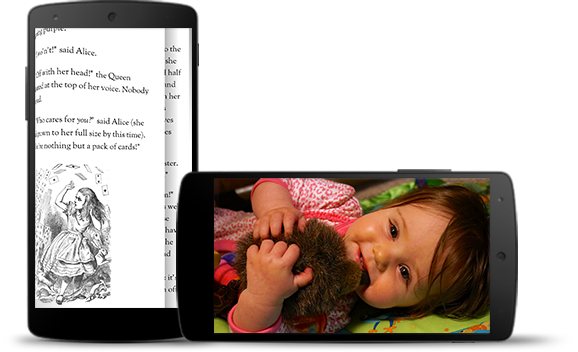
La modalità immersiva aiuta gli utenti a evitare uscite accidentali durante un gioco e offre un'esperienza immersiva per guardare immagini, video e libri. Tuttavia, tieni presente la frequenza con cui gli utenti aprono e chiudono le app per controllare le notifiche, eseguire ricerche improvvisate o intraprendere altre azioni. Poiché la modalità immersiva fa sì che gli utenti perdano l'accesso facile alla navigazione del sistema, utilizzala solo se il vantaggio per l'esperienza utente va oltre il semplice utilizzo di uno spazio sullo schermo aggiuntivo.
Usa WindowInsetsControllerCompat.hide()
per nascondere le barre di sistema e WindowInsetsControllerCompat.show()
per ripristinarle.
Lo snippet seguente mostra un esempio di configurazione di un pulsante per nascondere e mostrare le barre di sistema.
Kotlin
override fun onCreate(savedInstanceState: Bundle?) { ... val windowInsetsController = WindowCompat.getInsetsController(window, window.decorView) // Configure the behavior of the hidden system bars. windowInsetsController.systemBarsBehavior = WindowInsetsControllerCompat.BEHAVIOR_SHOW_TRANSIENT_BARS_BY_SWIPE // Add a listener to update the behavior of the toggle fullscreen button when // the system bars are hidden or revealed. ViewCompat.setOnApplyWindowInsetsListener(window.decorView) { view, windowInsets -> // You can hide the caption bar even when the other system bars are visible. // To account for this, explicitly check the visibility of navigationBars() // and statusBars() rather than checking the visibility of systemBars(). if (windowInsets.isVisible(WindowInsetsCompat.Type.navigationBars()) || windowInsets.isVisible(WindowInsetsCompat.Type.statusBars())) { binding.toggleFullscreenButton.setOnClickListener { // Hide both the status bar and the navigation bar. windowInsetsController.hide(WindowInsetsCompat.Type.systemBars()) } } else { binding.toggleFullscreenButton.setOnClickListener { // Show both the status bar and the navigation bar. windowInsetsController.show(WindowInsetsCompat.Type.systemBars()) } } ViewCompat.onApplyWindowInsets(view, windowInsets) } }
Java
@Override protected void onCreate(Bundle savedInstanceState) { ... WindowInsetsControllerCompat windowInsetsController = WindowCompat.getInsetsController(getWindow(), getWindow().getDecorView()); // Configure the behavior of the hidden system bars. windowInsetsController.setSystemBarsBehavior( WindowInsetsControllerCompat.BEHAVIOR_SHOW_TRANSIENT_BARS_BY_SWIPE ); // Add a listener to update the behavior of the toggle fullscreen button when // the system bars are hidden or revealed. ViewCompat.setOnApplyWindowInsetsListener( getWindow().getDecorView(), (view, windowInsets) -> { // You can hide the caption bar even when the other system bars are visible. // To account for this, explicitly check the visibility of navigationBars() // and statusBars() rather than checking the visibility of systemBars(). if (windowInsets.isVisible(WindowInsetsCompat.Type.navigationBars()) || windowInsets.isVisible(WindowInsetsCompat.Type.statusBars())) { binding.toggleFullscreenButton.setOnClickListener(v -> { // Hide both the status bar and the navigation bar. windowInsetsController.hide(WindowInsetsCompat.Type.systemBars()); }); } else { binding.toggleFullscreenButton.setOnClickListener(v -> { // Show both the status bar and the navigation bar. windowInsetsController.show(WindowInsetsCompat.Type.systemBars()); }); } return ViewCompat.onApplyWindowInsets(view, windowInsets); }); }
Se vuoi, puoi specificare il tipo di barre di sistema da nascondere e determinare il loro comportamento quando un utente interagisce con esse.
Specifica le barre di sistema da nascondere
Per specificare il tipo di barre di sistema da nascondere, passa uno dei seguenti parametri
a WindowInsetsControllerCompat.hide()
.
Usa
WindowInsetsCompat.Type.systemBars()
per nascondere entrambe le barre di sistema.Usa
WindowInsetsCompat.Type.statusBars()
per nascondere solo la barra di stato.Usa
WindowInsetsCompat.Type.navigationBars()
per nascondere solo la barra di navigazione.
Specifica il comportamento delle barre di sistema nascoste
Utilizza WindowInsetsControllerCompat.setSystemBarsBehavior()
per specificare il comportamento delle barre di sistema nascoste quando l'utente interagisce con esse.
Utilizza
WindowInsetsControllerCompat.BEHAVIOR_SHOW_BARS_BY_TOUCH
per visualizzare le barre di sistema nascoste a qualsiasi interazione dell'utente sul display corrispondente.Usa
WindowInsetsControllerCompat.BEHAVIOR_SHOW_BARS_BY_SWIPE
per visualizzare le barre di sistema nascoste con qualsiasi gesto di sistema, ad esempio scorrendo dal bordo dello schermo in cui è nascosta la barra.Usa
WindowInsetsControllerCompat.BEHAVIOR_SHOW_TRANSIENT_BARS_BY_SWIPE
per mostrare temporaneamente le barre di sistema nascoste con i gesti di sistema, ad esempio scorri dal bordo dello schermo in cui è nascosta la barra. Queste barre di sistema transitorie sovrappongono i contenuti dell'app, potrebbero avere un certo grado di trasparenza e vengono nascoste automaticamente dopo un breve timeout.