ส่วนต่อไปนี้จะอธิบายวิธีสร้างวิดเจ็ตแอปแบบง่ายด้วย "ข้อมูลโดยย่อ"
ประกาศ AppWidget
ในไฟล์ Manifest
หลังจากทำตามขั้นตอนการตั้งค่าแล้ว ให้ประกาศ AppWidget
และแท็ก
ข้อมูลเมตาในแอปของคุณ
- ลงทะเบียนผู้ให้บริการวิดเจ็ตแอปในไฟล์
AndroidManifest.xml
และไฟล์ข้อมูลเมตาที่เกี่ยวข้อง:
<receiver android:name=".glance.MyReceiver"
android:exported="true">
<intent-filter>
<action android:name="android.appwidget.action.APPWIDGET_UPDATE" />
</intent-filter>
<meta-data
android:name="android.appwidget.provider"
android:resource="@xml/my_app_widget_info" />
</receiver>
- ขยายตัวรับ
AppWidget
จากGlanceAppWidgetReceiver
:
class MyAppWidgetReceiver : GlanceAppWidgetReceiver() { override val glanceAppWidget: GlanceAppWidget = TODO("Create GlanceAppWidget") }
เพิ่มข้อมูลเมตา AppWidgetProviderInfo
ขั้นตอนถัดไป ให้ทำตามขั้นตอนนี้เพื่อเพิ่มข้อมูลเมตา AppWidgetProviderInfo
ทำตามคำแนะนำสร้างวิดเจ็ตอย่างง่ายเพื่อสร้างและกำหนดแอป ข้อมูลวิดเจ็ตในไฟล์
@xml/my_app_widget_info
ความแตกต่างเพียงอย่างเดียวสําหรับข้อมูลโดยย่อคือไม่มี
initialLayout
XML แต่ คุณต้องกำหนดอย่างหนึ่ง คุณสามารถใช้เลย์เอาต์การโหลดที่กำหนดไว้ล่วงหน้าซึ่งมีอยู่ใน ไลบรารี ได้แก่
<appwidget-provider xmlns:android="http://schemas.android.com/apk/res/android"
android:initialLayout="@layout/glance_default_loading_layout">
</appwidget-provider>
นิยามคำว่า GlanceAppWidget
สร้างชั้นเรียนใหม่ที่ขยายจาก
GlanceAppWidget
และลบล้างprovideGlance
นี่คือวิธีที่คุณสามารถโหลดข้อมูล ที่จำเป็นต่อการแสดงผลวิดเจ็ต
class MyAppWidget : GlanceAppWidget() { override suspend fun provideGlance(context: Context, id: GlanceId) { // In this method, load data needed to render the AppWidget. // Use `withContext` to switch to another thread for long running // operations. provideContent { // create your AppWidget here Text("Hello World") } } }
- สร้างอินสแตนซ์ใน
glanceAppWidget
บนGlanceAppWidgetReceiver
:
class MyAppWidgetReceiver : GlanceAppWidgetReceiver() { // Let MyAppWidgetReceiver know which GlanceAppWidget to use override val glanceAppWidget: GlanceAppWidget = MyAppWidget() }
ตอนนี้คุณกําหนดค่า AppWidget
โดยใช้ข้อมูลโดยย่อแล้ว
สร้าง UI
ข้อมูลโค้ดต่อไปนี้แสดงวิธีสร้าง UI
/* Import Glance Composables In the event there is a name clash with the Compose classes of the same name, you may rename the imports per https://kotlinlang.org/docs/packages.html#imports using the `as` keyword. import androidx.glance.Button import androidx.glance.layout.Column import androidx.glance.layout.Row import androidx.glance.text.Text */ class MyAppWidget : GlanceAppWidget() { override suspend fun provideGlance(context: Context, id: GlanceId) { // Load data needed to render the AppWidget. // Use `withContext` to switch to another thread for long running // operations. provideContent { // create your AppWidget here GlanceTheme { MyContent() } } } @Composable private fun MyContent() { Column( modifier = GlanceModifier.fillMaxSize() .background(GlanceTheme.colors.background), verticalAlignment = Alignment.Top, horizontalAlignment = Alignment.CenterHorizontally ) { Text(text = "Where to?", modifier = GlanceModifier.padding(12.dp)) Row(horizontalAlignment = Alignment.CenterHorizontally) { Button( text = "Home", onClick = actionStartActivity<MyActivity>() ) Button( text = "Work", onClick = actionStartActivity<MyActivity>() ) } } } }
ตัวอย่างโค้ดที่อยู่ก่อนหน้าดำเนินการดังต่อไปนี้
- ในระดับบนสุด
Column
ระบบจะวางรายการในแนวตั้งหลังแต่ละรายการ อื่นๆ Column
จะขยายขนาดให้ตรงกับพื้นที่ว่าง (ผ่านGlanceModifier
และจัดเนื้อหาให้อยู่ด้านบนสุด (verticalAlignment
) และอยู่กึ่งกลางแนวนอน (horizontalAlignment
)- เนื้อหาของ
Column
กำหนดโดยใช้ lambda การเรียงลำดับเป็นเรื่องสำคัญ
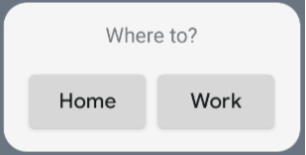
คุณสามารถเปลี่ยนค่าการจัดข้อความหรือใช้ค่าตัวปรับแต่งที่ต่างออกไป (เช่น ระยะห่างจากขอบ) เพื่อเปลี่ยนตำแหน่งและขนาดของคอมโพเนนต์ ดูข้อมูลอ้างอิง สำหรับรายการคอมโพเนนต์ พารามิเตอร์ และคอมโพเนนต์ทั้งหมด สำหรับแต่ละคลาส