إن القدرة على تحديد الأشكال المراد رسمها في سياق عرض OpenGL ES هي الخطوة الأولى في إنشاء رسومات متطورة لتطبيقك. يمكن أن يكون الرسم باستخدام OpenGL ES صعبًا بعض الشيء بدون عرفنا بعض الأشياء الأساسية عن الطريقة التي يتوقع منك بها OpenGL ES تعريف الكائنات الرسومية.
يشرح هذا الدرس نظام إحداثيات OpenGL ES الخاص بشاشة جهاز Android، أساسيات تحديد الشكل وشكل الوجوه، بالإضافة إلى تحديد المثلث والمربع.
تحديد المثلث
يتيح لك OpenGL ES تحديد الكائنات المرسومة باستخدام الإحداثيات في المساحة ثلاثية الأبعاد. وبالتالي،
قبل أن تتمكن من رسم مثلث، يجب تحديد إحداثياته. في OpenGL، الطريقة المعتادة لإجراء
لتحديد صفيف رأسي لأرقام النقطة العائمة للإحداثيات. بالنسبة إلى الحد الأقصى
الكفاءة، فأنت تكتب هذه الإحداثيات في ByteBuffer
، والذي يتم تمريره إلى
مسار رسومات OpenGL ES قيد المعالجة.
Kotlin
// number of coordinates per vertex in this array const val COORDS_PER_VERTEX = 3 var triangleCoords = floatArrayOf( // in counterclockwise order: 0.0f, 0.622008459f, 0.0f, // top -0.5f, -0.311004243f, 0.0f, // bottom left 0.5f, -0.311004243f, 0.0f // bottom right ) class Triangle { // Set color with red, green, blue and alpha (opacity) values val color = floatArrayOf(0.63671875f, 0.76953125f, 0.22265625f, 1.0f) private var vertexBuffer: FloatBuffer = // (number of coordinate values * 4 bytes per float) ByteBuffer.allocateDirect(triangleCoords.size * 4).run { // use the device hardware's native byte order order(ByteOrder.nativeOrder()) // create a floating point buffer from the ByteBuffer asFloatBuffer().apply { // add the coordinates to the FloatBuffer put(triangleCoords) // set the buffer to read the first coordinate position(0) } } }
Java
public class Triangle { private FloatBuffer vertexBuffer; // number of coordinates per vertex in this array static final int COORDS_PER_VERTEX = 3; static float triangleCoords[] = { // in counterclockwise order: 0.0f, 0.622008459f, 0.0f, // top -0.5f, -0.311004243f, 0.0f, // bottom left 0.5f, -0.311004243f, 0.0f // bottom right }; // Set color with red, green, blue and alpha (opacity) values float color[] = { 0.63671875f, 0.76953125f, 0.22265625f, 1.0f }; public Triangle() { // initialize vertex byte buffer for shape coordinates ByteBuffer bb = ByteBuffer.allocateDirect( // (number of coordinate values * 4 bytes per float) triangleCoords.length * 4); // use the device hardware's native byte order bb.order(ByteOrder.nativeOrder()); // create a floating point buffer from the ByteBuffer vertexBuffer = bb.asFloatBuffer(); // add the coordinates to the FloatBuffer vertexBuffer.put(triangleCoords); // set the buffer to read the first coordinate vertexBuffer.position(0); } }
يفترض OpenGL ES بشكل افتراضي نظام إحداثي حيث تحدد [0,0,0] (X,Y,Z) مركز
إطار GLSurfaceView
،
[1,1,0] هو الزاوية اليمنى العليا من الإطار
[-1,-1,0] هو الركن السفلي الأيسر من الإطار. للحصول على رسم توضيحي لنظام الإحداثيات هذا، راجع
مطوّر برامج OpenGL ES
الدليل.
لاحظ أن إحداثيات هذا الشكل يتم تحديدها بترتيب عكس عقارب الساعة. الرسم الترتيب مهمًا لأنه يحدد الجانب الأمامي للشكل، والذي عادةً ما تريد رسمها، والواجهة الخلفية، التي يمكنك اختيار عدم رسمها باستخدام خيار OpenGL ES ميزات الوجه. لمزيد من المعلومات عن الوجوه وطريقة الانتقاء، راجع OpenGL ES دليل المطوِّر.
تعريف مربع
يُعد تحديد المثلّثات أمرًا سهلاً للغاية في OpenGL، ولكن ماذا لو أردت الحصول على معقدة؟ لنفترض، مربع؟ وهناك عدة طرق للقيام بذلك، ولكن المسار النموذجي لرسم في OpenGL ES هو استخدام مثلثين مرسومين معًا:
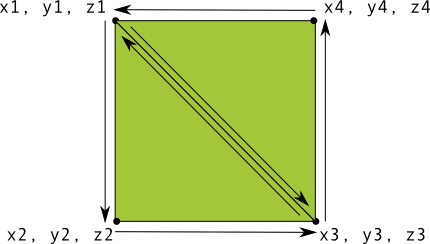
الشكل 1. رسم مربّع باستخدام مثلّثَين
مرة أخرى، يجب تحديد الرؤوس بترتيب عكس عقارب الساعة لكلا المثلثين اللذين
وتمثل هذا الشكل، وتضع القيم في ByteBuffer
. من أجل تجنب
لتحديد الإحداثيات التي يتشاركها كل مثلث مرتين، واستخدم قائمة رسم لمعرفة
برنامج رسومات OpenGL ES كيفية رسم هذه الرؤوس. إليك رمز هذا الشكل:
Kotlin
// number of coordinates per vertex in this array const val COORDS_PER_VERTEX = 3 var squareCoords = floatArrayOf( -0.5f, 0.5f, 0.0f, // top left -0.5f, -0.5f, 0.0f, // bottom left 0.5f, -0.5f, 0.0f, // bottom right 0.5f, 0.5f, 0.0f // top right ) class Square2 { private val drawOrder = shortArrayOf(0, 1, 2, 0, 2, 3) // order to draw vertices // initialize vertex byte buffer for shape coordinates private val vertexBuffer: FloatBuffer = // (# of coordinate values * 4 bytes per float) ByteBuffer.allocateDirect(squareCoords.size * 4).run { order(ByteOrder.nativeOrder()) asFloatBuffer().apply { put(squareCoords) position(0) } } // initialize byte buffer for the draw list private val drawListBuffer: ShortBuffer = // (# of coordinate values * 2 bytes per short) ByteBuffer.allocateDirect(drawOrder.size * 2).run { order(ByteOrder.nativeOrder()) asShortBuffer().apply { put(drawOrder) position(0) } } }
Java
public class Square { private FloatBuffer vertexBuffer; private ShortBuffer drawListBuffer; // number of coordinates per vertex in this array static final int COORDS_PER_VERTEX = 3; static float squareCoords[] = { -0.5f, 0.5f, 0.0f, // top left -0.5f, -0.5f, 0.0f, // bottom left 0.5f, -0.5f, 0.0f, // bottom right 0.5f, 0.5f, 0.0f }; // top right private short drawOrder[] = { 0, 1, 2, 0, 2, 3 }; // order to draw vertices public Square() { // initialize vertex byte buffer for shape coordinates ByteBuffer bb = ByteBuffer.allocateDirect( // (# of coordinate values * 4 bytes per float) squareCoords.length * 4); bb.order(ByteOrder.nativeOrder()); vertexBuffer = bb.asFloatBuffer(); vertexBuffer.put(squareCoords); vertexBuffer.position(0); // initialize byte buffer for the draw list ByteBuffer dlb = ByteBuffer.allocateDirect( // (# of coordinate values * 2 bytes per short) drawOrder.length * 2); dlb.order(ByteOrder.nativeOrder()); drawListBuffer = dlb.asShortBuffer(); drawListBuffer.put(drawOrder); drawListBuffer.position(0); } }
يمنحك هذا المثال نظرة خاطفة على ما يتطلبه الأمر لإنشاء أشكال أكثر تعقيدًا باستخدام OpenGL. ضِمن بشكل عام، يمكنك استخدام مجموعات من المثلثات لرسم الكائنات. في الدرس التالي، ستتعلم كيفية رسم هذه الأشكال على الشاشة.