每個文字欄位都需要輸入特定類型的文字,例如電子郵件地址、電話號碼或純文字。您必須為應用程式中的每個文字欄位指定輸入類型,讓系統顯示適當的軟輸入法,例如螢幕小鍵盤。
除了提供輸入法的按鈕類型之外,您還可以指定行為,例如輸入法是否提供拼字建議、將新句子大寫,並將車庫返回按鈕替換成動作按鈕 (例如「Done」或「Next」)。本頁說明如何指定這些特性。
指定鍵盤類型
一律在 <EditText>
元素中新增 android:inputType
屬性,藉此宣告文字欄位的輸入法。
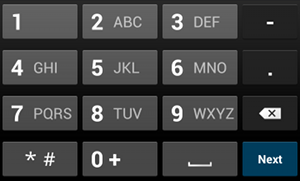
phone
輸入類型。舉例來說,如果您想透過輸入法輸入電話號碼,請使用 "phone"
值:
<EditText android:id="@+id/phone" android:layout_width="fill_parent" android:layout_height="wrap_content" android:hint="@string/phone_hint" android:inputType="phone" />
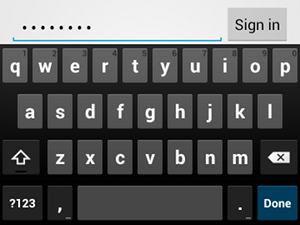
textPassword
輸入類型。如果文字欄位是密碼,請使用 "textPassword"
值,讓文字欄位讀出使用者輸入內容:
<EditText android:id="@+id/password" android:hint="@string/password_hint" android:inputType="textPassword" ... />
android:inputType
屬性記錄了幾個可能的值,您可以結合其中幾個值,指定輸入法的外觀和其他行為。
啟用拼字建議和其他行為
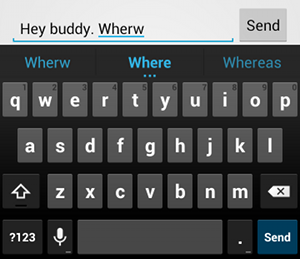
textAutoCorrect
即可自動更正錯字。android:inputType
屬性可讓您為輸入法指定各種行為。最重要的是,如果您的文字欄位適合用於基本文字輸入內容 (例如文字訊息),請使用 "textAutoCorrect"
值啟用自動拼字校正功能。
您可以使用 android:inputType
屬性結合不同行為和輸入法樣式。例如,以下說明如何建立將句子第一個字詞大寫的文字欄位,同時自動更正錯別字:
<EditText android:id="@+id/message" android:layout_width="wrap_content" android:layout_height="wrap_content" android:inputType= "textCapSentences|textAutoCorrect" ... />
指定輸入法動作
大多數的軟輸入法會在底部角落提供使用者動作按鈕,適用於目前的文字欄位。根據預設,除非文字欄位支援多行文字 (例如使用 android:inputType="textMultiLine"
),否則系統預設會將這個按鈕用於「Next」或「Done」動作。在這種情況下,動作按鈕為回車字元。不過,您可以指定可能更適合文字欄位的其他動作,例如「Send」或「Go」。
如要指定鍵盤動作按鈕,請使用 android:imeOptions
屬性搭配 "actionSend"
或 "actionSearch"
等動作值。例如:

android:imeOptions="actionSend"
時,系統會顯示「Send」按鈕。<EditText android:id="@+id/search" android:layout_width="fill_parent" android:layout_height="wrap_content" android:hint="@string/search_hint" android:inputType="text" android:imeOptions="actionSend" />
接著,您可以透過定義 EditText
元素的 TextView.OnEditorActionListener
,監聽動作按鈕的按下動作。在事件監聽器中回應 EditorInfo
類別中定義的適當輸入法編輯器動作 ID,例如 IME_ACTION_SEND
,如以下範例所示:
Kotlin
findViewById<EditText>(R.id.search).setOnEditorActionListener { v, actionId, event -> return@setOnEditorActionListener when (actionId) { EditorInfo.IME_ACTION_SEND -> { sendMessage() true } else -> false } }
Java
EditText editText = (EditText) findViewById(R.id.search); editText.setOnEditorActionListener(new OnEditorActionListener() { @Override public boolean onEditorAction(TextView v, int actionId, KeyEvent event) { boolean handled = false; if (actionId == EditorInfo.IME_ACTION_SEND) { sendMessage(); handled = true; } return handled; } });
提供自動完成建議
如要在使用者輸入內容時提供建議,您可以使用名為 AutoCompleteTextView
的 EditText
子類別。如要實作自動完成功能,您必須指定提供文字建議的 Adapter
。視資料的來源 (例如資料庫或陣列) 而定,您可以使用幾種轉接器。
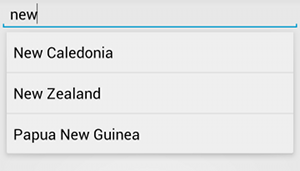
AutoCompleteTextView
範例。下列程序說明如何設定 AutoCompleteTextView
,以使用 ArrayAdapter
從陣列提供建議:
- 在版面配置中加入
AutoCompleteTextView
。以下是只包含文字欄位的版面配置:<?xml version="1.0" encoding="utf-8"?> <AutoCompleteTextView xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/autocomplete_country" android:layout_width="fill_parent" android:layout_height="wrap_content" />
- 定義包含所有文字建議的陣列。舉例來說,以下是國家/地區名稱陣列:
<?xml version="1.0" encoding="utf-8"?> <resources> <string-array name="countries_array"> <item>Afghanistan</item> <item>Albania</item> <item>Algeria</item> <item>American Samoa</item> <item>Andorra</item> <item>Angola</item> <item>Anguilla</item> <item>Antarctica</item> ... </string-array> </resources>
- 在
Activity
或Fragment
中,使用下列程式碼指定提供建議的轉換介面:Kotlin
// Get a reference to the AutoCompleteTextView in the layout. val textView = findViewById(R.id.autocomplete_country) as AutoCompleteTextView // Get the string array. val countries: Array<out String> = resources.getStringArray(R.array.countries_array) // Create the adapter and set it to the AutoCompleteTextView. ArrayAdapter<String>(this, android.R.layout.simple_list_item_1, countries).also { adapter -> textView.setAdapter(adapter) }
Java
// Get a reference to the AutoCompleteTextView in the layout. AutoCompleteTextView textView = (AutoCompleteTextView) findViewById(R.id.autocomplete_country); // Get the string array. String[] countries = getResources().getStringArray(R.array.countries_array); // Create the adapter and set it to the AutoCompleteTextView. ArrayAdapter<String> adapter = new ArrayAdapter<String>(this, android.R.layout.simple_list_item_1, countries); textView.setAdapter(adapter);
在上述範例中,系統會初始化新的
ArrayAdapter
,將countries_array
字串陣列中的每個項目繫結至simple_list_item_1
版面配置中的TextView
。這是 Android 提供的版面配置,為清單中的文字提供標準外觀。 -
呼叫
setAdapter()
,將轉接器指派給AutoCompleteTextView
。