セグメント化されたボタンを使用すると、ユーザーがオプションのセットから並べて選択できます。セグメント化されたボタンには次の 2 種類があります。
- 単一選択ボタン: ユーザーが 1 つのオプションを選択できるようにします。
- 複数選択ボタン: ユーザーが 2 ~ 5 個のアイテムを選択できるようにします。選択肢が複雑な場合や、アイテムが 5 つを超える場合は、チップを使用します。
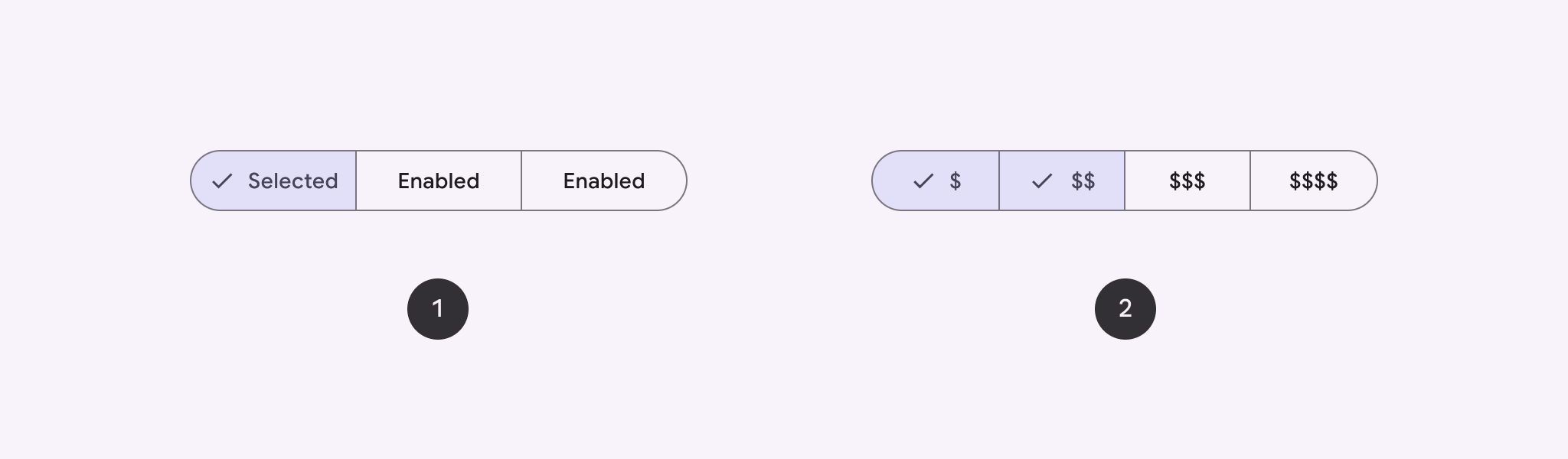
API サーフェス
セグメント化されたボタンを作成するには、SingleChoiceSegmentedButtonRow
レイアウトと MultiChoiceSegmentedButtonRow
レイアウトを使用します。これらのレイアウトでは、SegmentedButton
の配置とサイズが正しく行われるようにし、次の主なパラメータを共有します。
space
: ボタン間の重なりを調整します。content
: セグメント化されたボタン行のコンテンツが含まれます。通常はSegmentedButton
のシーケンスです。
単一選択のセグメント化されたボタンを作成する
次の例は、単一選択のセグメント化されたボタンを作成する方法を示しています。
@Composable fun SingleChoiceSegmentedButton(modifier: Modifier = Modifier) { var selectedIndex by remember { mutableIntStateOf(0) } val options = listOf("Day", "Month", "Week") SingleChoiceSegmentedButtonRow { options.forEachIndexed { index, label -> SegmentedButton( shape = SegmentedButtonDefaults.itemShape( index = index, count = options.size ), onClick = { selectedIndex = index }, selected = index == selectedIndex, label = { Text(label) } ) } } }
コードに関する主なポイント
remember
とmutableIntStateOf
を使用してselectedIndex
変数を初期化し、現在選択されているボタンのインデックスを追跡します。- ボタンラベルを表す
options
のリストを定義します。 SingleChoiceSegmentedButtonRow
を使用すると、選択できるボタンは 1 つだけになります。forEachIndexed
ループ内に、オプションごとにSegmentedButton
を作成します。shape
は、インデックスとSegmentedButtonDefaults.itemShape
を使用したオプションの合計数に基づいて、ボタンの形状を定義します。onClick
は、クリックされたボタンのインデックスでselectedIndex
を更新します。selected
は、selectedIndex
に基づいてボタンの選択状態を設定します。label
は、Text
コンポーザブルを使用してボタンラベルを表示します。
結果
![[日]、[月]、[週] のオプションが付いたセグメント化されたボタン コンポーネントが表示されます。現在、[日] オプションが選択されています。](https://developer.android.google.cn/static/develop/ui/compose/images/components/SingleChoiceSegmentedButton.png?hl=ja)
複数選択セグメント ボタンを作成する
この例では、ユーザーが複数のオプションを選択できるように、アイコン付きの多選択セグメント化ボタンを作成する方法を示します。
@Composable fun MultiChoiceSegmentedButton(modifier: Modifier = Modifier) { val selectedOptions = remember { mutableStateListOf(false, false, false) } val options = listOf("Walk", "Ride", "Drive") MultiChoiceSegmentedButtonRow { options.forEachIndexed { index, label -> SegmentedButton( shape = SegmentedButtonDefaults.itemShape( index = index, count = options.size ), checked = selectedOptions[index], onCheckedChange = { selectedOptions[index] = !selectedOptions[index] }, icon = { SegmentedButtonDefaults.Icon(selectedOptions[index]) }, label = { when (label) { "Walk" -> Icon( imageVector = Icons.AutoMirrored.Filled.DirectionsWalk, contentDescription = "Directions Walk" ) "Ride" -> Icon( imageVector = Icons.Default.DirectionsBus, contentDescription = "Directions Bus" ) "Drive" -> Icon( imageVector = Icons.Default.DirectionsCar, contentDescription = "Directions Car" ) } } ) } } }
コードに関する主なポイント
- このコードは、
remember
とmutableStateListOf
を使用してselectedOptions
変数を初期化します。これにより、各ボタンの選択状態が追跡されます。 - このコードでは、
MultiChoiceSegmentedButtonRow
を使用してボタンを格納しています。 forEachIndexed
ループ内で、コードはオプションごとにSegmentedButton
を作成します。shape
は、インデックスとオプションの合計数に基づいてボタンの形状を定義します。checked
は、selectedOptions
の対応する値に基づいてボタンのチェック状態を設定します。onCheckedChange
は、ボタンがクリックされたときに、selectedOptions
内の対応するアイテムの選択状態を切り替えます。icon
は、SegmentedButtonDefaults.Icon
とボタンのチェック状態に基づいてアイコンを表示します。label
は、適切な画像ベクトルとコンテンツの説明を含むIcon
コンポーザブルを使用して、ラベルに対応するアイコンを表示します。
結果
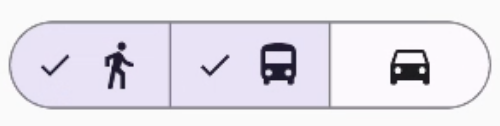
参考情報
- マテリアル 3: セグメント化されたボタン