Compose では、ポリゴンからシェイプを作成できます。たとえば、次の種類のシェイプを作成できます。
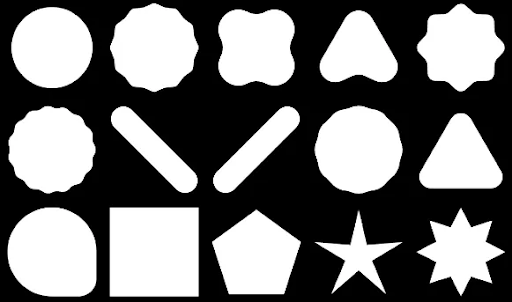
Compose でカスタムの丸みを帯びたポリゴンを作成するには、app/build.gradle
に graphics-shapes
依存関係を追加します。
implementation "androidx.graphics:graphics-shapes:1.0.0-alpha05"
このライブラリを使用すると、ポリゴンからシェイプを作成できます。多角形の角は直線で、角が鋭いだけですが、これらの形では必要に応じて角を丸くすることもできます。2 つの異なる形状に簡単にモーフィングできます。任意の形状間のモーフィングは困難であり、設計時に問題となる傾向があります。このライブラリでは、類似した多角形構造を持つこれらのシェイプ間でモーフィングを行うことで、簡素化が実現しています。
ポリゴンを作成する
次のスニペットでは、描画領域の中心に 6 つのポイントがある基本のポリゴン図形が作成されます。
Box( modifier = Modifier .drawWithCache { val roundedPolygon = RoundedPolygon( numVertices = 6, radius = size.minDimension / 2, centerX = size.width / 2, centerY = size.height / 2 ) val roundedPolygonPath = roundedPolygon.toPath().asComposePath() onDrawBehind { drawPath(roundedPolygonPath, color = Color.Blue) } } .fillMaxSize() )
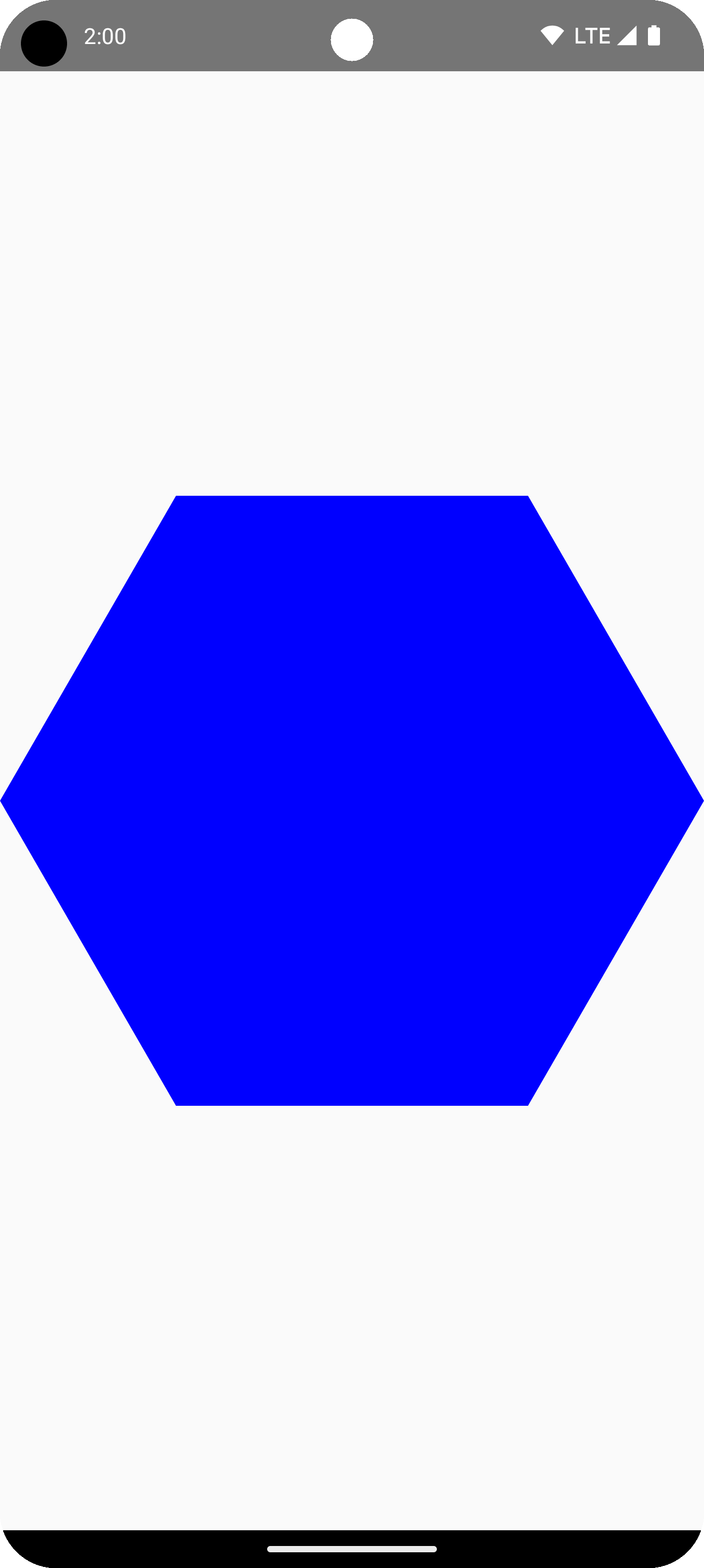
この例では、ライブラリは、リクエストされたシェイプを表すジオメトリを保持する RoundedPolygon
を作成します。Compose アプリでそのシェイプを描画するには、そこから Path
オブジェクトを取得して、Compose が描画方法を認識するフォームにシェイプを変換する必要があります。
多角形の角を丸くする
ポリゴンの角を丸くするには、CornerRounding
パラメータを使用します。これには、radius
と smoothing
の 2 つのパラメータを指定します。丸い隅は 1 ~ 3 の 3 次曲線で構成されます。その中心は円弧を描き、2 つの側面(「側面」)は形状のエッジから中心曲線に移行します。
Radius
radius
は、頂点を丸くするために使用される円の半径です。
たとえば、次のように角の丸い三角形を作成します。
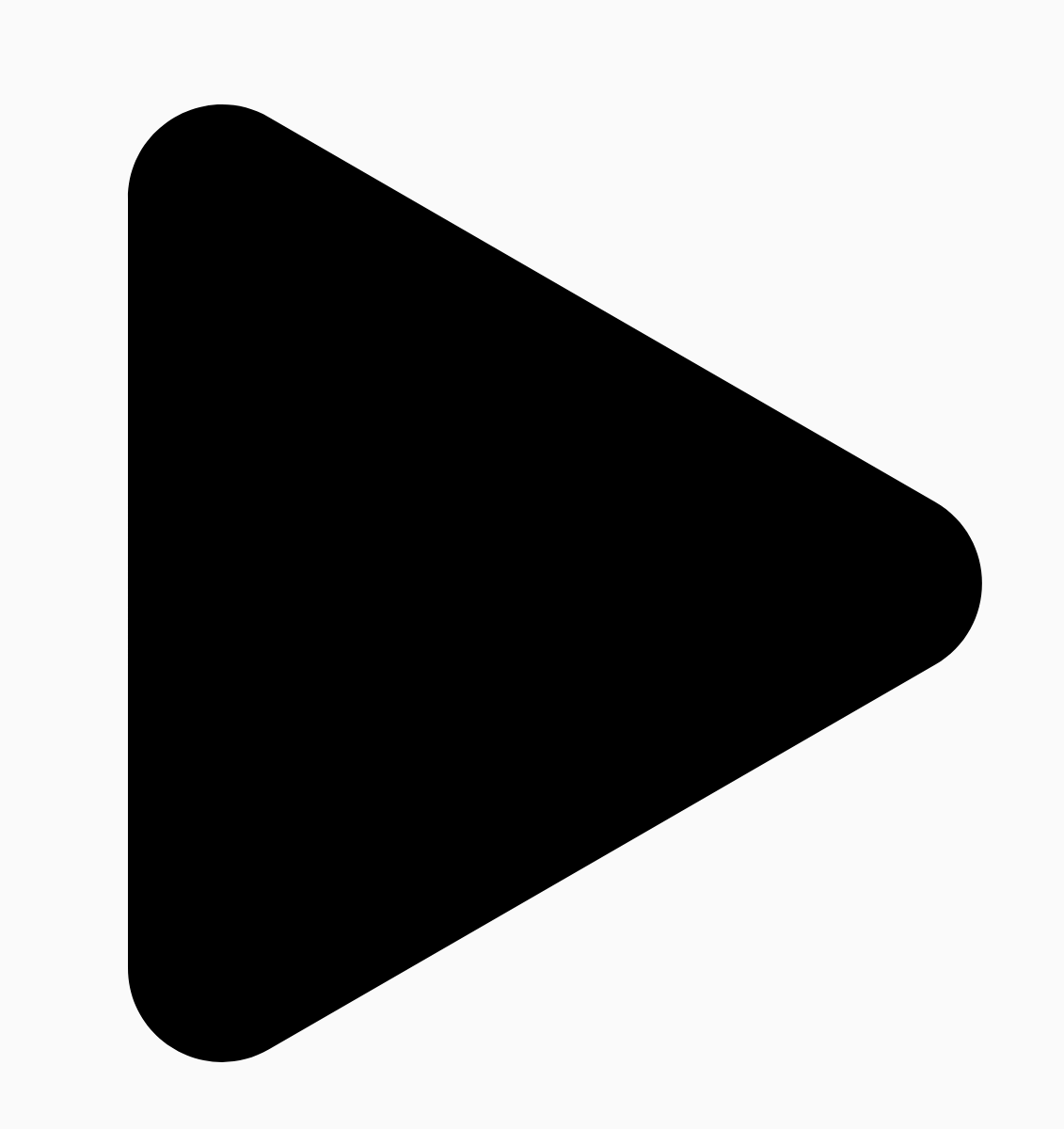
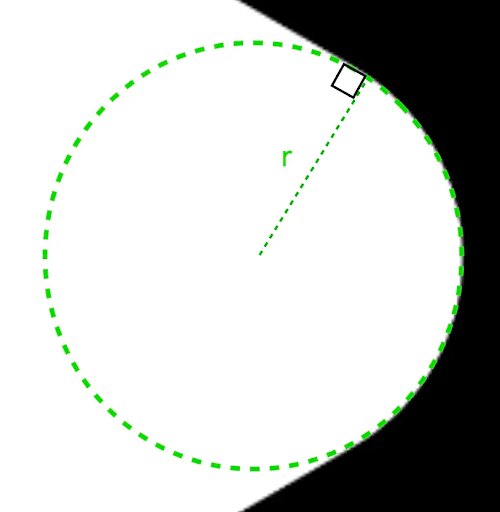
r
によって、角の丸い丸みのサイズが決まります。スムージング
平滑化は、角の丸い部分から端までにかかる時間を決定する要素です。平滑化係数が 0(平滑化されていない、CornerRounding
のデフォルト値)の場合、純粋に円形の角丸になります。平滑化係数が 0 以外(最大 1.0)の場合、角が 3 つの別々の曲線で丸められます。
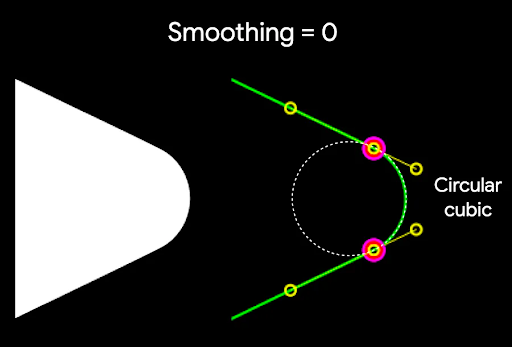
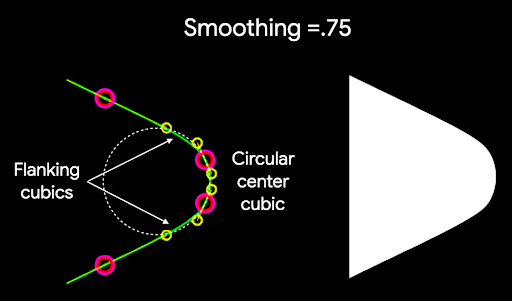
たとえば、以下のスニペットは、平滑化を 0 に設定した場合と 1 に設定した場合の微妙な違いを示しています。
Box( modifier = Modifier .drawWithCache { val roundedPolygon = RoundedPolygon( numVertices = 3, radius = size.minDimension / 2, centerX = size.width / 2, centerY = size.height / 2, rounding = CornerRounding( size.minDimension / 10f, smoothing = 0.1f ) ) val roundedPolygonPath = roundedPolygon.toPath().asComposePath() onDrawBehind { drawPath(roundedPolygonPath, color = Color.Black) } } .size(100.dp) )
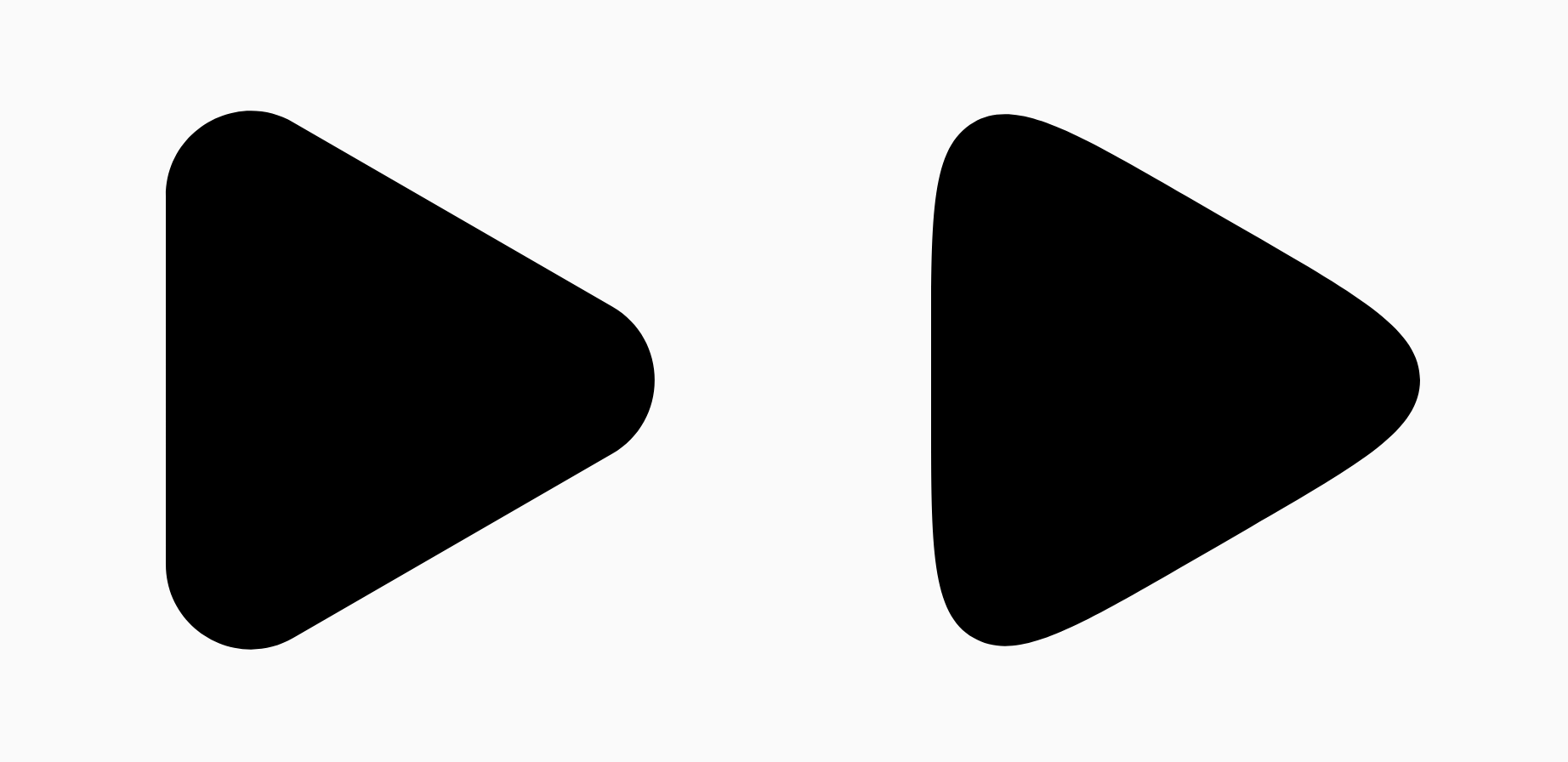
サイズと位置
デフォルトでは、シェイプは中心(0, 0
)を中心とする半径 1
で作成されます。この半径は、シェイプのベースとなるポリゴンの中心から外側の頂点までの距離を表します。角を丸くすると、頂点よりも角が丸くなって中心に近いため、形状が小さくなります。ポリゴンのサイズを調整するには、radius
の値を調整します。位置を調整するには、ポリゴンの centerX
または centerY
を変更します。または、DrawScope#translate()
などの標準の DrawScope
変換関数を使用して、サイズ、位置、回転を変更するようにオブジェクトを変換します。
変形形状
Morph
オブジェクトは、2 つの多角形間のアニメーションを表す新しいシェイプです。2 つのシェイプ間でモーフィングするには、2 つの RoundedPolygons
オブジェクトと、この 2 つのシェイプを取る Morph
オブジェクトを作成します。開始シェイプと終了シェイプの間のシェイプを計算するには、0 ~ 1 の progress
値を指定して、開始(0)と終了(1)のシェイプの間のフォームを決定します。
Box( modifier = Modifier .drawWithCache { val triangle = RoundedPolygon( numVertices = 3, radius = size.minDimension / 2f, centerX = size.width / 2f, centerY = size.height / 2f, rounding = CornerRounding( size.minDimension / 10f, smoothing = 0.1f ) ) val square = RoundedPolygon( numVertices = 4, radius = size.minDimension / 2f, centerX = size.width / 2f, centerY = size.height / 2f ) val morph = Morph(start = triangle, end = square) val morphPath = morph .toPath(progress = 0.5f).asComposePath() onDrawBehind { drawPath(morphPath, color = Color.Black) } } .fillMaxSize() )
上記の例では、2 つのシェイプ(角の丸い三角形と正方形)のちょうど中間であり、次の結果になります。
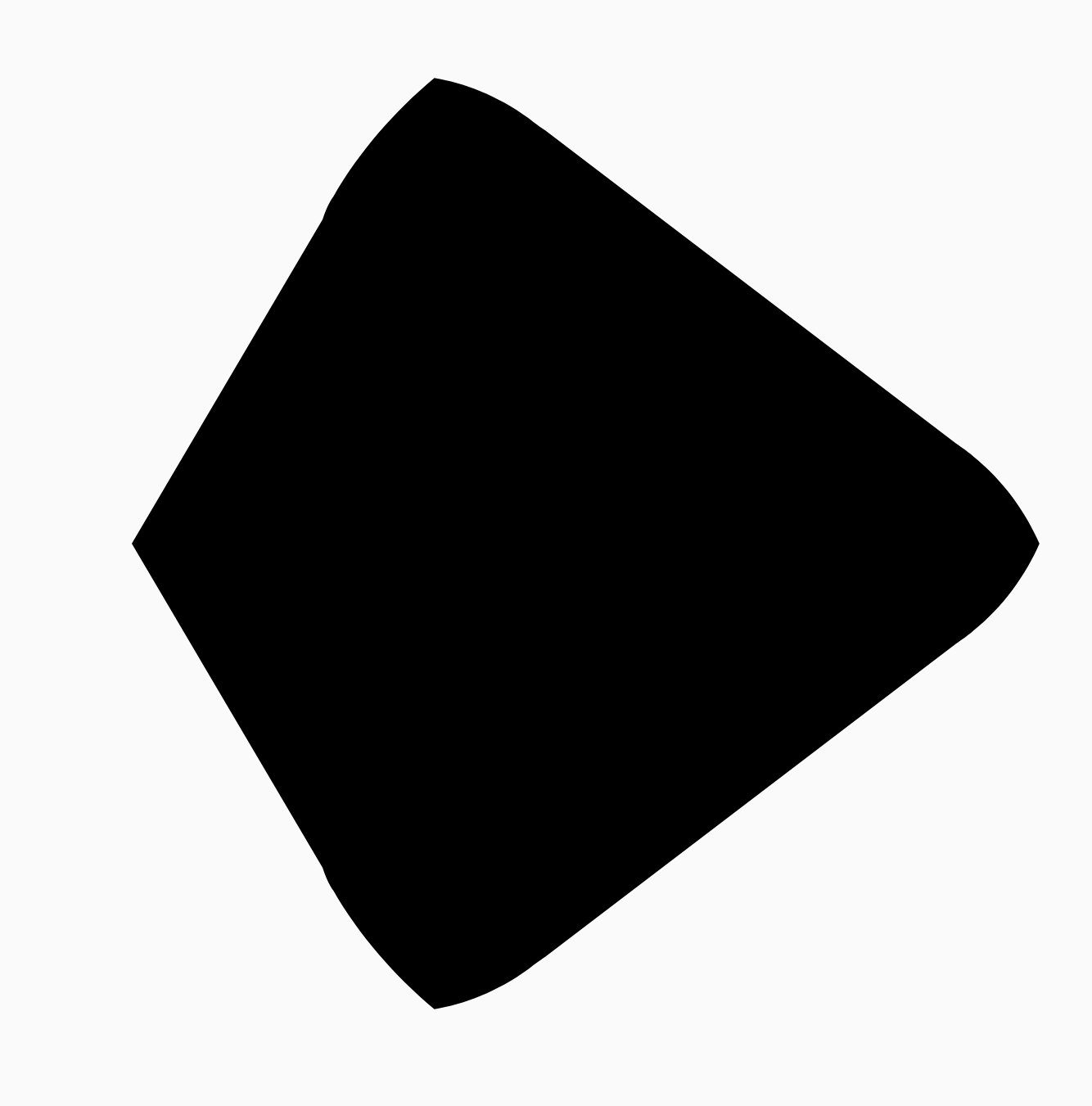
ほとんどのシナリオでは、モーフィングは単なる静的レンダリングではなく、アニメーションの一部として行われます。これら 2 つの間をアニメーション化するには、標準の Compose のアニメーション API を使用して、進行状況の値を時間の経過とともに変更します。たとえば、次のように、この 2 つのシェイプの間でモーフを無限にアニメーション化できます。
val infiniteAnimation = rememberInfiniteTransition(label = "infinite animation") val morphProgress = infiniteAnimation.animateFloat( initialValue = 0f, targetValue = 1f, animationSpec = infiniteRepeatable( tween(500), repeatMode = RepeatMode.Reverse ), label = "morph" ) Box( modifier = Modifier .drawWithCache { val triangle = RoundedPolygon( numVertices = 3, radius = size.minDimension / 2f, centerX = size.width / 2f, centerY = size.height / 2f, rounding = CornerRounding( size.minDimension / 10f, smoothing = 0.1f ) ) val square = RoundedPolygon( numVertices = 4, radius = size.minDimension / 2f, centerX = size.width / 2f, centerY = size.height / 2f ) val morph = Morph(start = triangle, end = square) val morphPath = morph .toPath(progress = morphProgress.value) .asComposePath() onDrawBehind { drawPath(morphPath, color = Color.Black) } } .fillMaxSize() )
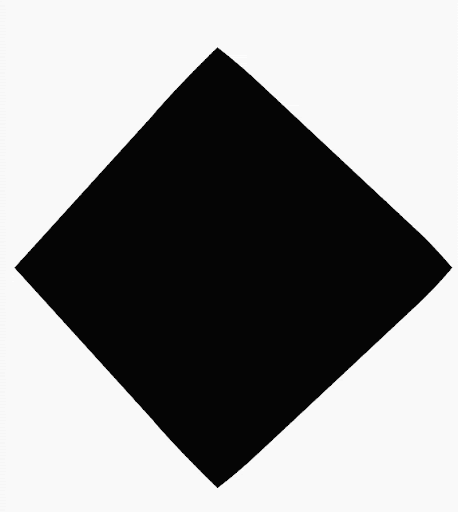
ポリゴンをクリップとして使用
Compose で clip
修飾子を使用してコンポーザブルのレンダリング方法を変更し、クリッピング領域の周囲を描画するシャドウを利用するのが一般的です。
fun RoundedPolygon.getBounds() = calculateBounds().let { Rect(it[0], it[1], it[2], it[3]) } class RoundedPolygonShape( private val polygon: RoundedPolygon, private var matrix: Matrix = Matrix() ) : Shape { private var path = Path() override fun createOutline( size: Size, layoutDirection: LayoutDirection, density: Density ): Outline { path.rewind() path = polygon.toPath().asComposePath() matrix.reset() val bounds = polygon.getBounds() val maxDimension = max(bounds.width, bounds.height) matrix.scale(size.width / maxDimension, size.height / maxDimension) matrix.translate(-bounds.left, -bounds.top) path.transform(matrix) return Outline.Generic(path) } }
次のスニペットに示すように、ポリゴンをクリップとして使用できます。
val hexagon = remember { RoundedPolygon( 6, rounding = CornerRounding(0.2f) ) } val clip = remember(hexagon) { RoundedPolygonShape(polygon = hexagon) } Box( modifier = Modifier .clip(clip) .background(MaterialTheme.colorScheme.secondary) .size(200.dp) ) { Text( "Hello Compose", color = MaterialTheme.colorScheme.onSecondary, modifier = Modifier.align(Alignment.Center) ) }
結果は次のようになります。
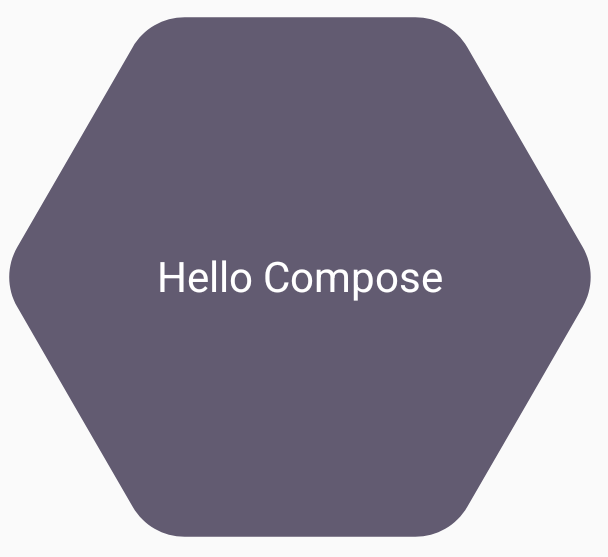
これは、以前のレンダリングとそれほど変わらないように見えるかもしれませんが、Compose の他の機能を活用できます。たとえば、次の方法を使用して画像をクリップし、クリップした領域の周囲にシャドウを適用できます。
val hexagon = remember { RoundedPolygon( 6, rounding = CornerRounding(0.2f) ) } val clip = remember(hexagon) { RoundedPolygonShape(polygon = hexagon) } Box( modifier = Modifier.fillMaxSize(), contentAlignment = Alignment.Center ) { Image( painter = painterResource(id = R.drawable.dog), contentDescription = "Dog", contentScale = ContentScale.Crop, modifier = Modifier .graphicsLayer { this.shadowElevation = 6.dp.toPx() this.shape = clip this.clip = true this.ambientShadowColor = Color.Black this.spotShadowColor = Color.Black } .size(200.dp) ) }
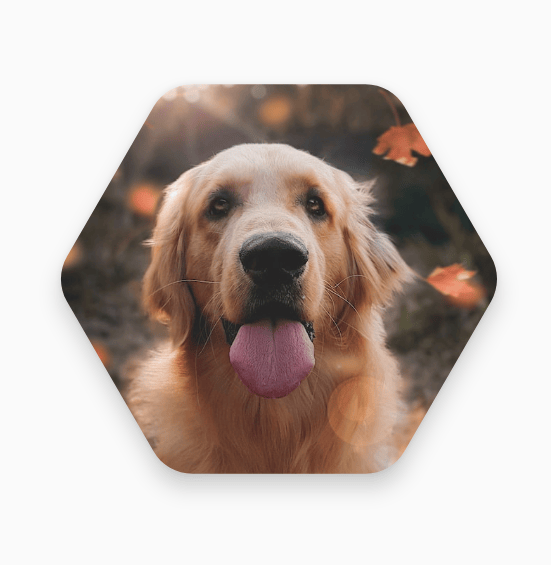
クリック時のモーフボタン
graphics-shape
ライブラリを使用して、押すと 2 つのシェイプ間でモーフィングするボタンを作成できます。まず、Shape
を拡張する MorphPolygonShape
を作成し、適切にサイズ調整および変換します。進行状況が渡されていることに注意してください。これにより、シェイプをアニメーション化できます。
class MorphPolygonShape( private val morph: Morph, private val percentage: Float ) : Shape { private val matrix = Matrix() override fun createOutline( size: Size, layoutDirection: LayoutDirection, density: Density ): Outline { // Below assumes that you haven't changed the default radius of 1f, nor the centerX and centerY of 0f // By default this stretches the path to the size of the container, if you don't want stretching, use the same size.width for both x and y. matrix.scale(size.width / 2f, size.height / 2f) matrix.translate(1f, 1f) val path = morph.toPath(progress = percentage).asComposePath() path.transform(matrix) return Outline.Generic(path) } }
このモーフ形状を使用するには、shapeA
と shapeB
の 2 つのポリゴンを作成します。Morph
を作成して記憶します。次に、ボタンを押すと、interactionSource
をアニメーションの駆動力として使用して、モーフをクリップの輪郭としてボタンに適用します。
val shapeA = remember { RoundedPolygon( 6, rounding = CornerRounding(0.2f) ) } val shapeB = remember { RoundedPolygon.star( 6, rounding = CornerRounding(0.1f) ) } val morph = remember { Morph(shapeA, shapeB) } val interactionSource = remember { MutableInteractionSource() } val isPressed by interactionSource.collectIsPressedAsState() val animatedProgress = animateFloatAsState( targetValue = if (isPressed) 1f else 0f, label = "progress", animationSpec = spring(dampingRatio = 0.4f, stiffness = Spring.StiffnessMedium) ) Box( modifier = Modifier .size(200.dp) .padding(8.dp) .clip(MorphPolygonShape(morph, animatedProgress.value)) .background(Color(0xFF80DEEA)) .size(200.dp) .clickable(interactionSource = interactionSource, indication = null) { } ) { Text("Hello", modifier = Modifier.align(Alignment.Center)) }
これにより、ボックスをタップすると次のようなアニメーションになります。
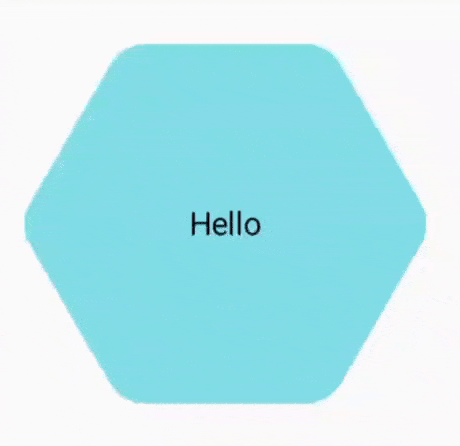
形状の変化を無限にアニメーション化する
モーフ形状を無限にアニメーション化するには、rememberInfiniteTransition
を使用します。以下は、時間の経過とともに形が無限に変化(回転)するプロフィール写真の例です。この方法では、上記の MorphPolygonShape
を少し調整します。
class CustomRotatingMorphShape( private val morph: Morph, private val percentage: Float, private val rotation: Float ) : Shape { private val matrix = Matrix() override fun createOutline( size: Size, layoutDirection: LayoutDirection, density: Density ): Outline { // Below assumes that you haven't changed the default radius of 1f, nor the centerX and centerY of 0f // By default this stretches the path to the size of the container, if you don't want stretching, use the same size.width for both x and y. matrix.scale(size.width / 2f, size.height / 2f) matrix.translate(1f, 1f) matrix.rotateZ(rotation) val path = morph.toPath(progress = percentage).asComposePath() path.transform(matrix) return Outline.Generic(path) } } @Preview @Composable private fun RotatingScallopedProfilePic() { val shapeA = remember { RoundedPolygon( 12, rounding = CornerRounding(0.2f) ) } val shapeB = remember { RoundedPolygon.star( 12, rounding = CornerRounding(0.2f) ) } val morph = remember { Morph(shapeA, shapeB) } val infiniteTransition = rememberInfiniteTransition("infinite outline movement") val animatedProgress = infiniteTransition.animateFloat( initialValue = 0f, targetValue = 1f, animationSpec = infiniteRepeatable( tween(2000, easing = LinearEasing), repeatMode = RepeatMode.Reverse ), label = "animatedMorphProgress" ) val animatedRotation = infiniteTransition.animateFloat( initialValue = 0f, targetValue = 360f, animationSpec = infiniteRepeatable( tween(6000, easing = LinearEasing), repeatMode = RepeatMode.Reverse ), label = "animatedMorphProgress" ) Box( modifier = Modifier.fillMaxSize(), contentAlignment = Alignment.Center ) { Image( painter = painterResource(id = R.drawable.dog), contentDescription = "Dog", contentScale = ContentScale.Crop, modifier = Modifier .clip( CustomRotatingMorphShape( morph, animatedProgress.value, animatedRotation.value ) ) .size(200.dp) ) } }
このコードでは、次のような興味深い結果が得られます。
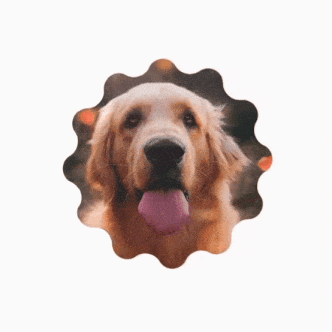
カスタムのポリゴン
正多角形から作成したシェイプがユースケースに合わない場合は、頂点のリストを使ってよりカスタムのシェイプを作成できます。たとえば、次のようなハートの形を作成できます。
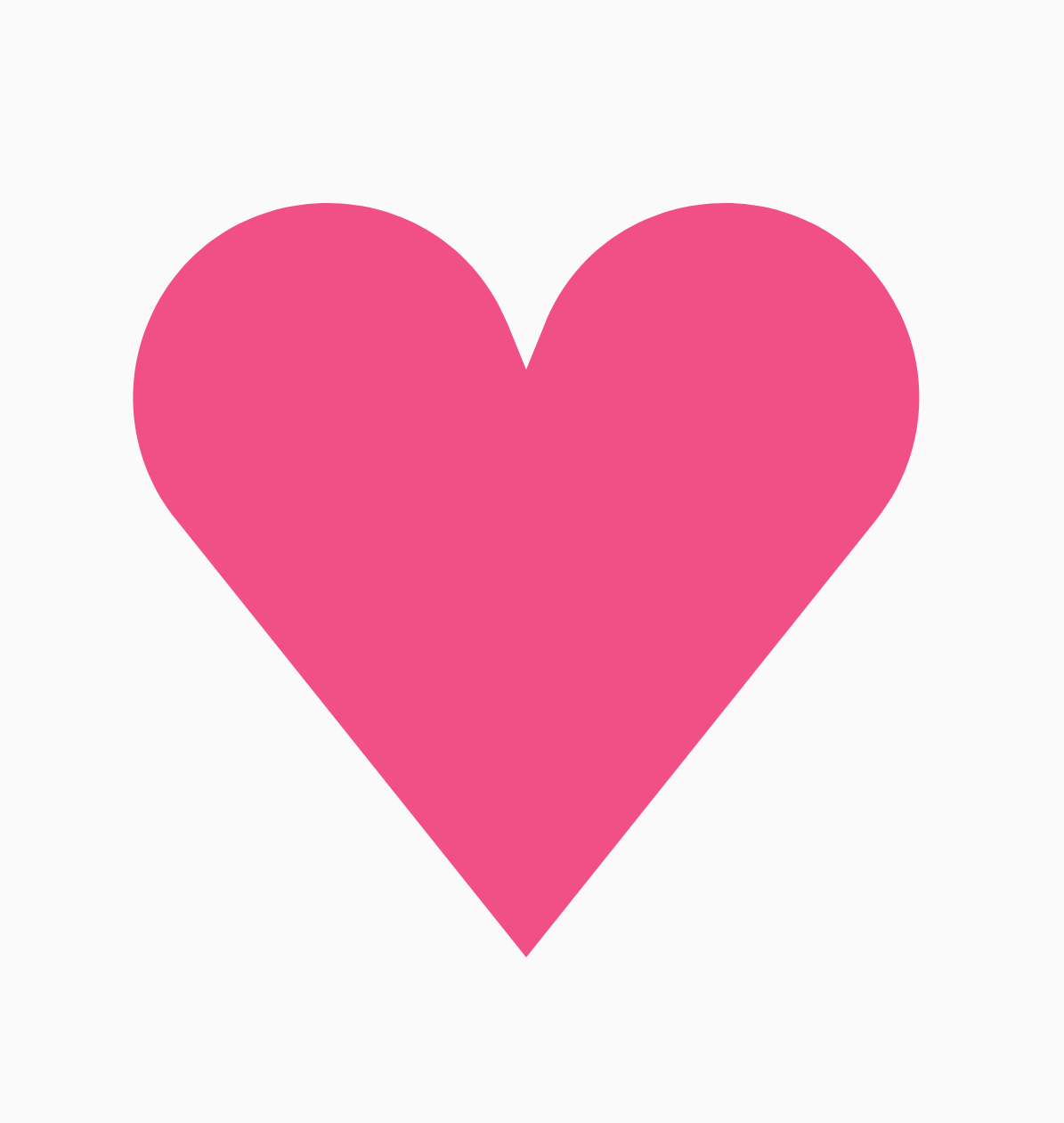
x 座標と y 座標の浮動小数点数の配列を受け取る RoundedPolygon
オーバーロードを使用して、このシェイプの個々の頂点を指定できます。
心臓のポリゴンを分割する場合、点を指定する極座標系の方がデカルト(x,y)座標系を使用するよりも簡単です。この場合、0°
は右側から始まり時計回りに進み、270°
は 12 時の位置になります。
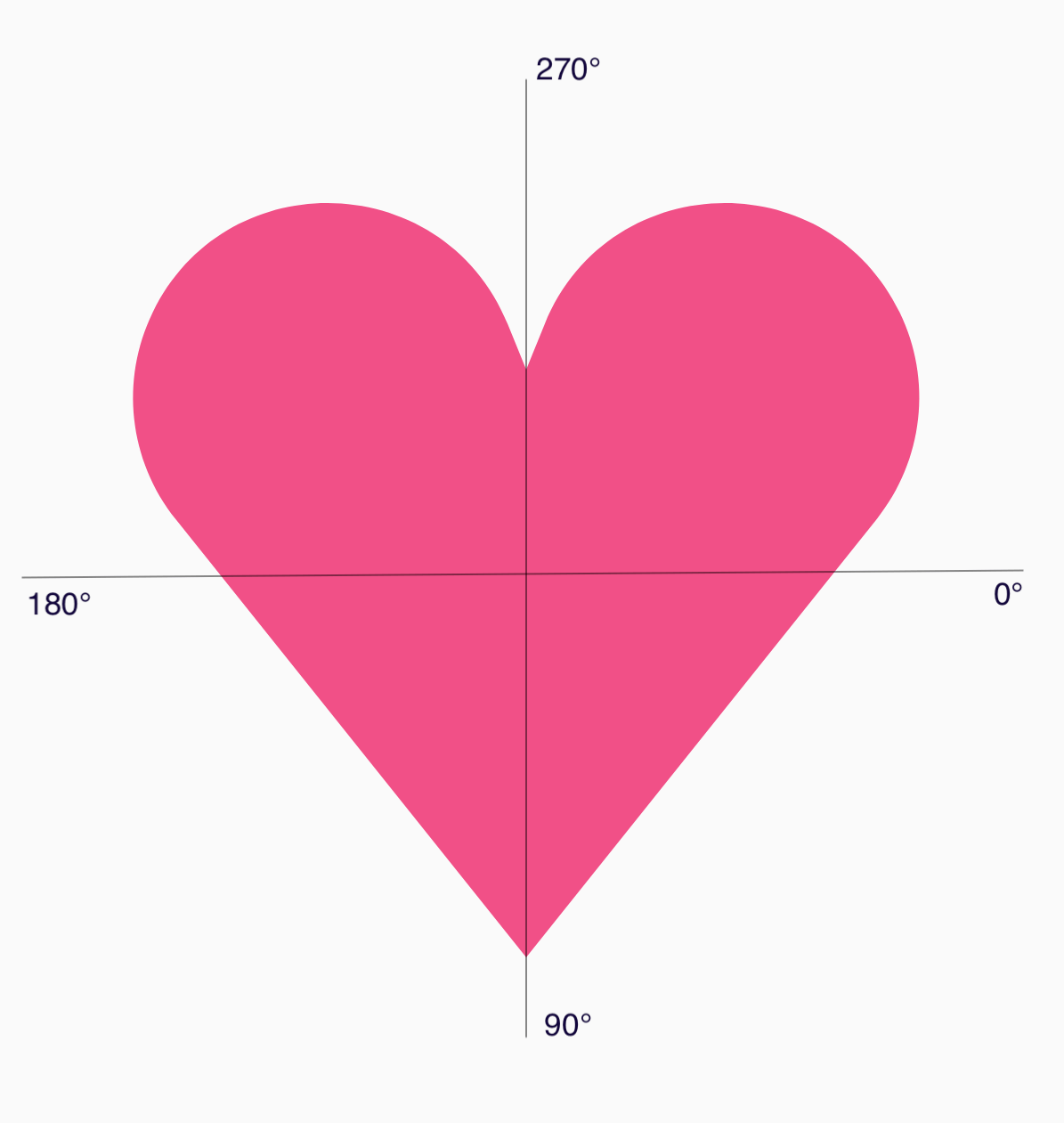
各点の中心からの角度(Θ)と半径を指定することで、より簡単にシェイプを定義できます。
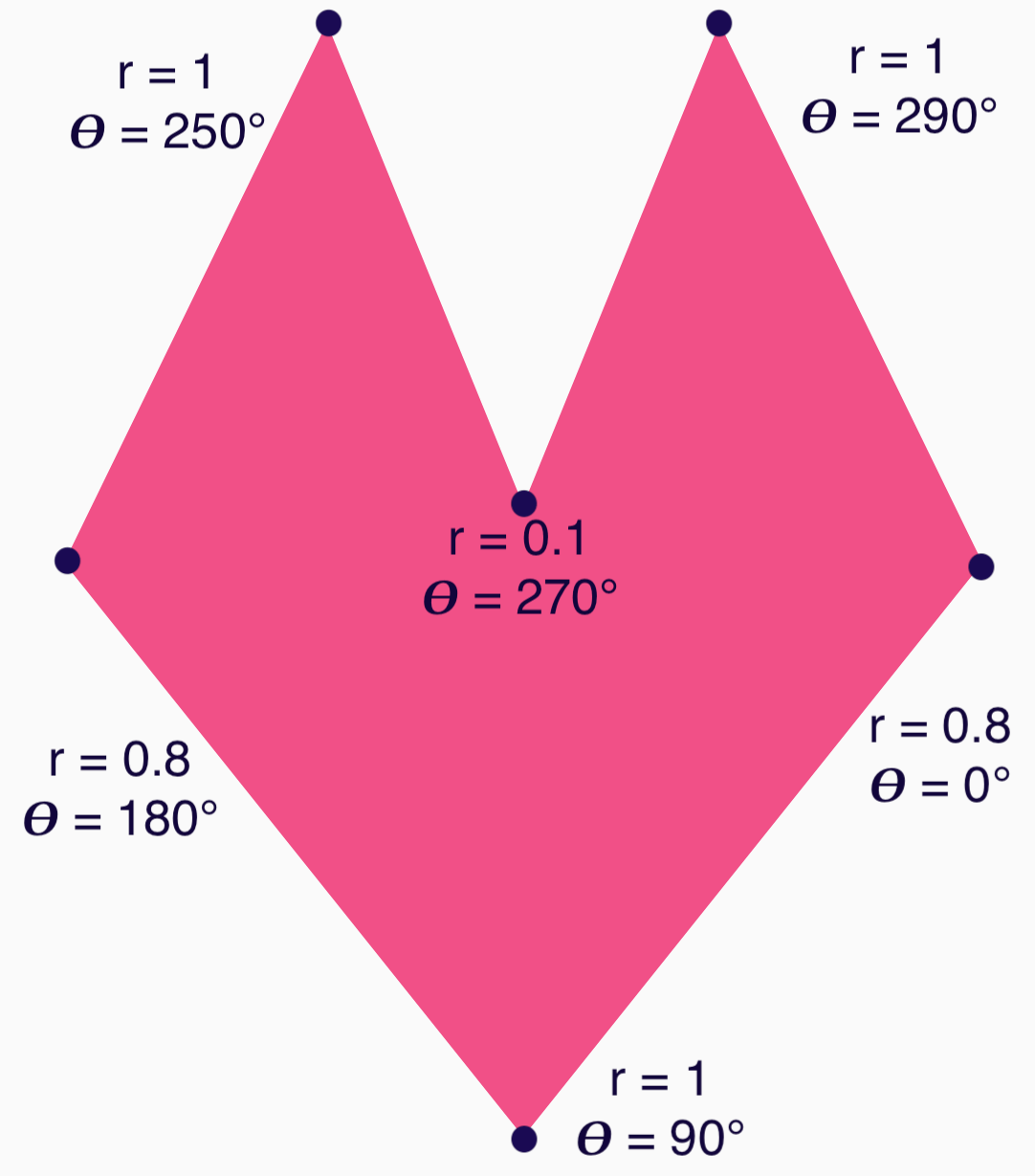
これで、頂点を作成して RoundedPolygon
関数に渡すことができます。
val vertices = remember { val radius = 1f val radiusSides = 0.8f val innerRadius = .1f floatArrayOf( radialToCartesian(radiusSides, 0f.toRadians()).x, radialToCartesian(radiusSides, 0f.toRadians()).y, radialToCartesian(radius, 90f.toRadians()).x, radialToCartesian(radius, 90f.toRadians()).y, radialToCartesian(radiusSides, 180f.toRadians()).x, radialToCartesian(radiusSides, 180f.toRadians()).y, radialToCartesian(radius, 250f.toRadians()).x, radialToCartesian(radius, 250f.toRadians()).y, radialToCartesian(innerRadius, 270f.toRadians()).x, radialToCartesian(innerRadius, 270f.toRadians()).y, radialToCartesian(radius, 290f.toRadians()).x, radialToCartesian(radius, 290f.toRadians()).y, ) }
次の radialToCartesian
関数を使用して、頂点をデカルト座標に変換する必要があります。
internal fun Float.toRadians() = this * PI.toFloat() / 180f internal val PointZero = PointF(0f, 0f) internal fun radialToCartesian( radius: Float, angleRadians: Float, center: PointF = PointZero ) = directionVectorPointF(angleRadians) * radius + center internal fun directionVectorPointF(angleRadians: Float) = PointF(cos(angleRadians), sin(angleRadians))
上記のコードでは、ハートの未加工の頂点が得られますが、選択したハートの形を取得するには、特定の角を丸くする必要があります。90°
と 270°
の角には丸みがなく、それ以外の角には丸みがあります。個々の角にカスタムの丸みを設定するには、perVertexRounding
パラメータを使用します。
val rounding = remember { val roundingNormal = 0.6f val roundingNone = 0f listOf( CornerRounding(roundingNormal), CornerRounding(roundingNone), CornerRounding(roundingNormal), CornerRounding(roundingNormal), CornerRounding(roundingNone), CornerRounding(roundingNormal), ) } val polygon = remember(vertices, rounding) { RoundedPolygon( vertices = vertices, perVertexRounding = rounding ) } Box( modifier = Modifier .drawWithCache { val roundedPolygonPath = polygon.toPath().asComposePath() onDrawBehind { scale(size.width * 0.5f, size.width * 0.5f) { translate(size.width * 0.5f, size.height * 0.5f) { drawPath(roundedPolygonPath, color = Color(0xFFF15087)) } } } } .size(400.dp) )
その結果、ピンク色のハートになります。
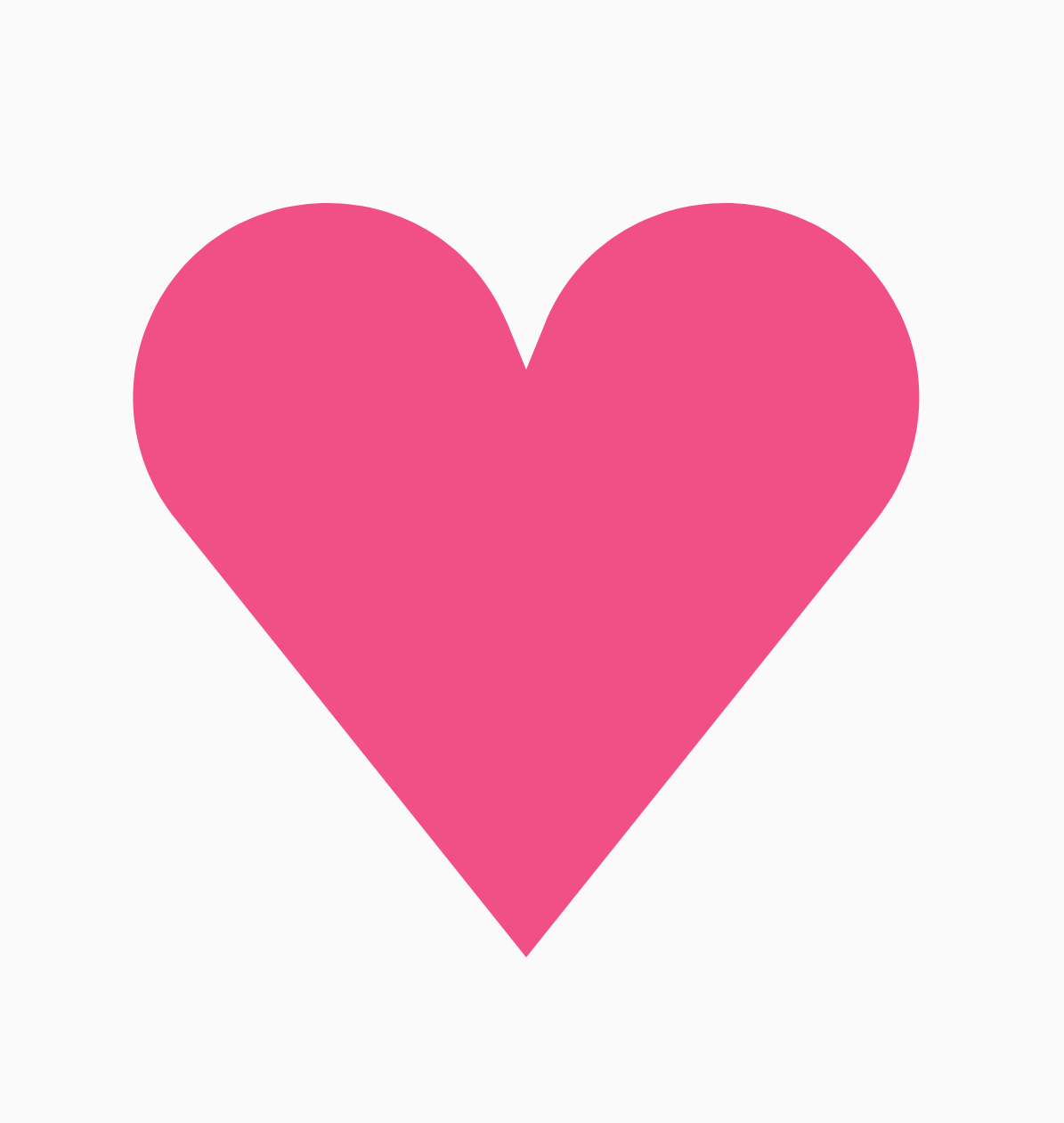
上記のシェイプがユースケースに適していない場合は、Path
クラスを使用してカスタム シェイプを描画するか、ディスクから ImageVector
ファイルを読み込むことを検討してください。graphics-shapes
ライブラリは、任意の形状に使用することは想定されていませんが、丸みを帯びたポリゴンの作成と、ポリゴン間のモーフィング アニメーションを簡素化することを目的としています。
参考情報
詳細と例については、次のリソースをご覧ください。