良好的视觉设计是应用成功的关键,而配色方案是设计的主要组成部分。Palette 库是一个 Jetpack 库,可以从图片中提取突出颜色,创建具有视觉吸引力的应用。
您可以使用 Palette 库设计布局主题背景,并将自定义颜色应用于应用中的视觉元素。例如,您可以使用调色板根据歌曲的专辑封面为歌曲创建颜色协调的片头字幕,或者在应用的背景图片发生变化时调整应用的工具栏颜色。通过 Palette
对象,您可以访问 Bitmap
图片中的颜色,同时还可以提供位图中的六个主要颜色配置文件,以便您选择设计。
设置调色板库
如需使用 Palette 库,请将以下内容添加到 build.gradle
:
Kotlin
android { compileSdkVersion(33) ... } dependencies { ... implementation("androidx.palette:palette:1.0.0") }
Groovy
android { compileSdkVersion 33 ... } dependencies { ... implementation 'androidx.palette:palette:1.0.0' }
创建调色板
借助 Palette
对象,您可以访问图片中的主要颜色以及叠加文本的相应颜色。您可以使用调色板来设计应用的样式,并根据给定的来源图片动态更改应用的配色方案。
如需创建调色板,请先从 Bitmap
实例化 Palette.Builder
。然后,您可以在生成调色板之前使用 Palette.Builder
对其进行自定义。本部分介绍如何通过位图图像生成调色板和自定义。
生成调色板实例
使用 from(Bitmap bitmap)
方法生成 Palette
实例,首先根据 Bitmap
创建 Palette.Builder
。
构建器可以同步或异步生成调色板。如果您想在被调用方法的同一线程上创建调色板,请使用同步生成调色板。如果您在另一个线程上异步生成调色板,请使用 onGenerated()
方法在创建调色板后立即访问该调色板。
以下代码段提供了这两种类型的调色板生成方法的示例方法:
Kotlin
// Generate palette synchronously and return it. fun createPaletteSync(bitmap: Bitmap): Palette = Palette.from(bitmap).generate() // Generate palette asynchronously and use it on a different thread using onGenerated(). fun createPaletteAsync(bitmap: Bitmap) { Palette.from(bitmap).generate { palette -> // Use generated instance. } }
Java
// Generate palette synchronously and return it. public Palette createPaletteSync(Bitmap bitmap) { Palette p = Palette.from(bitmap).generate(); return p; } // Generate palette asynchronously and use it on a different thread using onGenerated(). public void createPaletteAsync(Bitmap bitmap) { Palette.from(bitmap).generate(new PaletteAsyncListener() { public void onGenerated(Palette p) { // Use generated instance. } }); }
如果您需要为已排序的图片或对象列表连续生成调色板,请考虑缓存 Palette
实例以防止界面性能变慢。请勿在主线程上创建调色板。
自定义调色板
借助 Palette.Builder
,您可以通过选择生成的调色板中的颜色数量、构建器用于生成调色板的图片区域以及包含在调色板中的颜色来自定义调色板。例如,您可以滤除黑色,或确保构建器仅使用图片的上半部分来生成调色板。
使用 Palette.Builder
类中的以下方法微调调色板的大小和颜色:
addFilter()
- 此方法会添加一个过滤条件,用于指明生成的调色板中可以有哪些颜色。传入您自己的
Palette.Filter
并修改其isAllowed()
方法,以确定从调色板中滤除哪些颜色。 maximumColorCount()
- 此方法可用于设置调色板中的颜色数量上限。默认值为 16,最佳值取决于源图片。对于横向图片,最佳值范围为 8-16,而有人脸的照片值通常介于 24-32 之间。
Palette.Builder
需要更长的时间才能生成包含更多颜色的调色板。 setRegion()
- 此方法指示在创建调色板时生成工具使用的位图的区域。您只能在从位图生成调色板时使用此方法,而且这不会影响原始图片。
addTarget()
- 此方法允许您通过向构建器添加
Target
颜色配置文件来自行执行颜色匹配。如果默认Target
无法满足需求,高级开发者可以使用Target.Builder
创建自己的Target
。
提取颜色配置文件
根据 Material Design 的标准,Palette 库会从图片中提取常用的颜色配置文件。每个配置文件均由 Target
定义,并根据饱和度、亮度和总体(由颜色表示的位图中的像素数)针对每个配置文件对从位图图像中提取的颜色进行评分。对于每个配置文件,得分最高的颜色用于定义给定图片的颜色配置文件。
默认情况下,Palette
对象包含给定图片中的 16 种主要颜色。生成调色板时,您可以使用 Palette.Builder
自定义其颜色数量。提取更多颜色可以提高每个颜色配置文件匹配的可能性,但也会导致 Palette.Builder
在生成调色板时花费的时间更长。
Palette 库会尝试提取以下六种颜色配置文件:
- Light Vibrant
- Vibrant
- Dark Vibrant
- Light Muted
- Muted
- Dark Muted
Palette
中的每个 get<Profile>Color()
方法均返回与该特定配置文件相关联的调色板中的颜色,其中 <Profile>
会替换为六个颜色配置文件之一的名称。例如,获取 Dark Vibrant 颜色配置文件的方法是 getDarkVibrantColor()
。
由于并非所有图片都包含所有颜色配置文件,因此请提供默认颜色以返回。
图 1 显示了一张照片及其通过 get<Profile>Color()
方法获得的相应颜色配置文件。
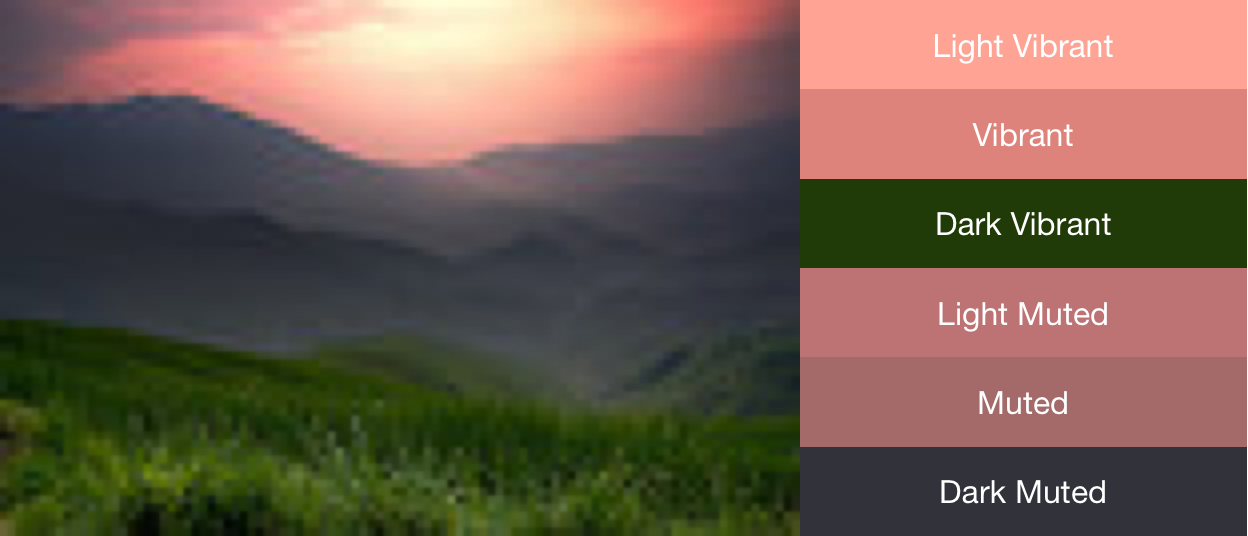
使用色样创建配色方案
Palette
类还会为每个颜色配置文件生成 Palette.Swatch
对象。Palette.Swatch
对象包含该配置文件的关联颜色以及颜色的填充(以像素为单位)。
色样提供了额外的方法来访问有关颜色配置文件的更多信息,例如 HSL 值和像素填充。借助 getBodyTextColor()
和 getTitleTextColor()
方法,您可以使用色样帮助创建更全面的配色方案和应用主题。这些方法会返回适合在色样颜色上使用的颜色。
Palette
中的每个 get<Profile>Swatch()
方法都会返回与该特定配置文件相关联的色样,其中 <Profile>
会替换为六种颜色配置文件之一的名称。虽然调色板的 get<Profile>Swatch()
方法不需要默认值参数,但如果图片中不存在该特定配置文件,则这些方法会返回 null
。因此,在使用色样之前,请检查确保色样不为 null。例如,如果“Vibrant”色样不为 null,以下代码会从调色板中获取标题文本颜色:
Kotlin
val vibrant = myPalette.vibrantSwatch // In Kotlin, check for null before accessing properties on the vibrant swatch. val titleColor = vibrant?.titleTextColor
Java
Palette.Swatch vibrant = myPalette.getVibrantSwatch(); if(vibrant != null){ int titleColor = vibrant.getTitleTextColor(); // ... }
如需访问调色板中的所有颜色,getSwatches()
方法会返回由图片生成的所有色样的列表,包括标准的六种颜色配置文件。
以下代码段使用上述代码段中的方法同步生成调色板,获取其鲜艳色样,并更改工具栏的颜色以匹配位图图像。图 2 显示了生成的图片和工具栏。
Kotlin
// Set the background and text colors of a toolbar given a bitmap image to // match. fun setToolbarColor(bitmap: Bitmap) { // Generate the palette and get the vibrant swatch. val vibrantSwatch = createPaletteSync(bitmap).vibrantSwatch // Set the toolbar background and text colors. // Fall back to default colors if the vibrant swatch isn't available. with(findViewById<Toolbar>(R.id.toolbar)) { setBackgroundColor(vibrantSwatch?.rgb ?: ContextCompat.getColor(context, R.color.default_title_background)) setTitleTextColor(vibrantSwatch?.titleTextColor ?: ContextCompat.getColor(context, R.color.default_title_color)) } }
Java
// Set the background and text colors of a toolbar given a bitmap image to // match. public void setToolbarColor(Bitmap bitmap) { // Generate the palette and get the vibrant swatch. // See the createPaletteSync() method from the preceding code snippet. Palette p = createPaletteSync(bitmap); Palette.Swatch vibrantSwatch = p.getVibrantSwatch(); Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar); // Load default colors. int backgroundColor = ContextCompat.getColor(getContext(), R.color.default_title_background); int textColor = ContextCompat.getColor(getContext(), R.color.default_title_color); // Check that the Vibrant swatch is available. if(vibrantSwatch != null){ backgroundColor = vibrantSwatch.getRgb(); textColor = vibrantSwatch.getTitleTextColor(); } // Set the toolbar background and text colors. toolbar.setBackgroundColor(backgroundColor); toolbar.setTitleTextColor(textColor); }
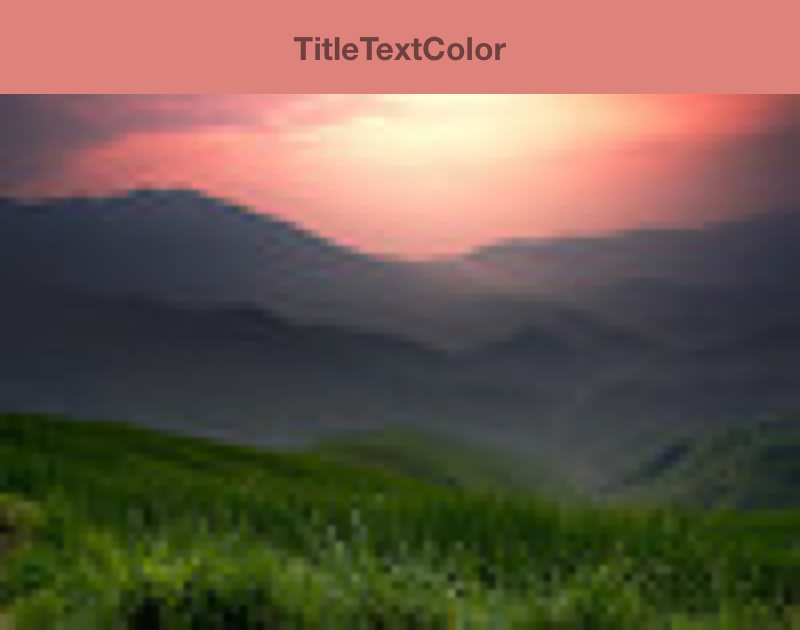