有些内容在全屏模式下可获得最佳体验,屏幕上没有显示任何指示器 状态栏或导航栏。例如视频、游戏、图片库、图书和演示文稿幻灯片。这称为 沉浸模式。本页将介绍如何利用 全屏显示的内容。
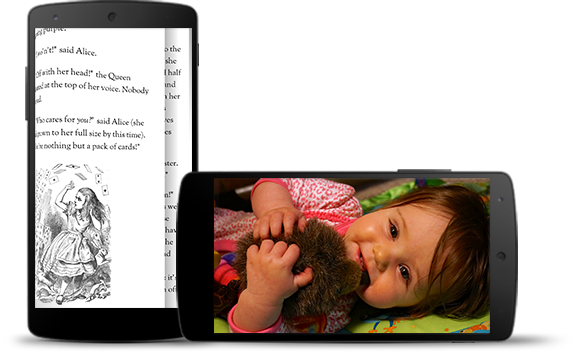
沉浸模式可帮助用户在游戏过程中避免意外退出,以及 为图片、视频和图书提供沉浸式体验。 不过,请注意用户进入和退出应用查看通知的频率, 进行随机搜索或执行其他操作。因为沉浸模式 导致用户无法轻松使用系统导航,请仅使用沉浸模式 对用户体验的好处不仅仅是使用额外的屏幕 空间。
使用 WindowInsetsControllerCompat.hide()
隐藏系统栏,使用 WindowInsetsControllerCompat.show()
调出系统栏。
以下代码段展示了将按钮配置为隐藏和显示的示例 系统栏。
override fun onCreate(savedInstanceState: Bundle?) { ... val windowInsetsController = WindowCompat.getInsetsController(window, window.decorView) // Configure the behavior of the hidden system bars. windowInsetsController.systemBarsBehavior = WindowInsetsControllerCompat.BEHAVIOR_SHOW_TRANSIENT_BARS_BY_SWIPE // Add a listener to update the behavior of the toggle fullscreen button when // the system bars are hidden or revealed. ViewCompat.setOnApplyWindowInsetsListener(window.decorView) { view, windowInsets -> // You can hide the caption bar even when the other system bars are visible. // To account for this, explicitly check the visibility of navigationBars() // and statusBars() rather than checking the visibility of systemBars(). if (windowInsets.isVisible(WindowInsetsCompat.Type.navigationBars()) || windowInsets.isVisible(WindowInsetsCompat.Type.statusBars())) { binding.toggleFullscreenButton.setOnClickListener { // Hide both the status bar and the navigation bar. windowInsetsController.hide(WindowInsetsCompat.Type.systemBars()) } } else { binding.toggleFullscreenButton.setOnClickListener { // Show both the status bar and the navigation bar. windowInsetsController.show(WindowInsetsCompat.Type.systemBars()) } } ViewCompat.onApplyWindowInsets(view, windowInsets) } }
@Override protected void onCreate(Bundle savedInstanceState) { ... WindowInsetsControllerCompat windowInsetsController = WindowCompat.getInsetsController(getWindow(), getWindow().getDecorView()); // Configure the behavior of the hidden system bars. windowInsetsController.setSystemBarsBehavior( WindowInsetsControllerCompat.BEHAVIOR_SHOW_TRANSIENT_BARS_BY_SWIPE ); // Add a listener to update the behavior of the toggle fullscreen button when // the system bars are hidden or revealed. ViewCompat.setOnApplyWindowInsetsListener( getWindow().getDecorView(), (view, windowInsets) -> { // You can hide the caption bar even when the other system bars are visible. // To account for this, explicitly check the visibility of navigationBars() // and statusBars() rather than checking the visibility of systemBars(). if (windowInsets.isVisible(WindowInsetsCompat.Type.navigationBars()) || windowInsets.isVisible(WindowInsetsCompat.Type.statusBars())) { binding.toggleFullscreenButton.setOnClickListener(v -> { // Hide both the status bar and the navigation bar. windowInsetsController.hide(WindowInsetsCompat.Type.systemBars()); }); } else { binding.toggleFullscreenButton.setOnClickListener(v -> { // Show both the status bar and the navigation bar. windowInsetsController.show(WindowInsetsCompat.Type.systemBars()); }); } return ViewCompat.onApplyWindowInsets(view, windowInsets); }); }
您可以选择指定要隐藏的系统栏的类型,并确定用户与系统栏互动时的行为。
指定要隐藏的系统栏
如需指定要隐藏的系统栏类型,请传递以下参数之一
发送至 WindowInsetsControllerCompat.hide()
。
使用
WindowInsetsCompat.Type.systemBars()
执行以下操作: 隐藏两个系统栏。使用
WindowInsetsCompat.Type.statusBars()
仅隐藏状态栏。使用
WindowInsetsCompat.Type.navigationBars()
执行以下操作: 仅隐藏导航栏。
指定隐藏系统栏的行为
使用 WindowInsetsControllerCompat.setSystemBarsBehavior()
指定隐藏的系统栏在用户与其互动时应如何表现。
使用
WindowInsetsControllerCompat.BEHAVIOR_SHOW_BARS_BY_TOUCH
显示隐藏的系统栏。 。使用
WindowInsetsControllerCompat.BEHAVIOR_SHOW_BARS_BY_SWIPE
可在执行任何系统手势(例如从隐藏系统栏的屏幕边缘滑动)时显示隐藏的系统栏。使用
WindowInsetsControllerCompat.BEHAVIOR_SHOW_TRANSIENT_BARS_BY_SWIPE
来暂时显示隐藏的系统栏。 从隐藏栏的屏幕边缘滑动。这些瞬时系统栏会叠加在应用内容之上,可能具有一定程度的透明度,并会在短暂的超时后自动隐藏。