幾乎所有的多裝置體驗都從尋找可用裝置開始。目的地: 要簡化這個一般工作,我們提供 Device Discovery API。
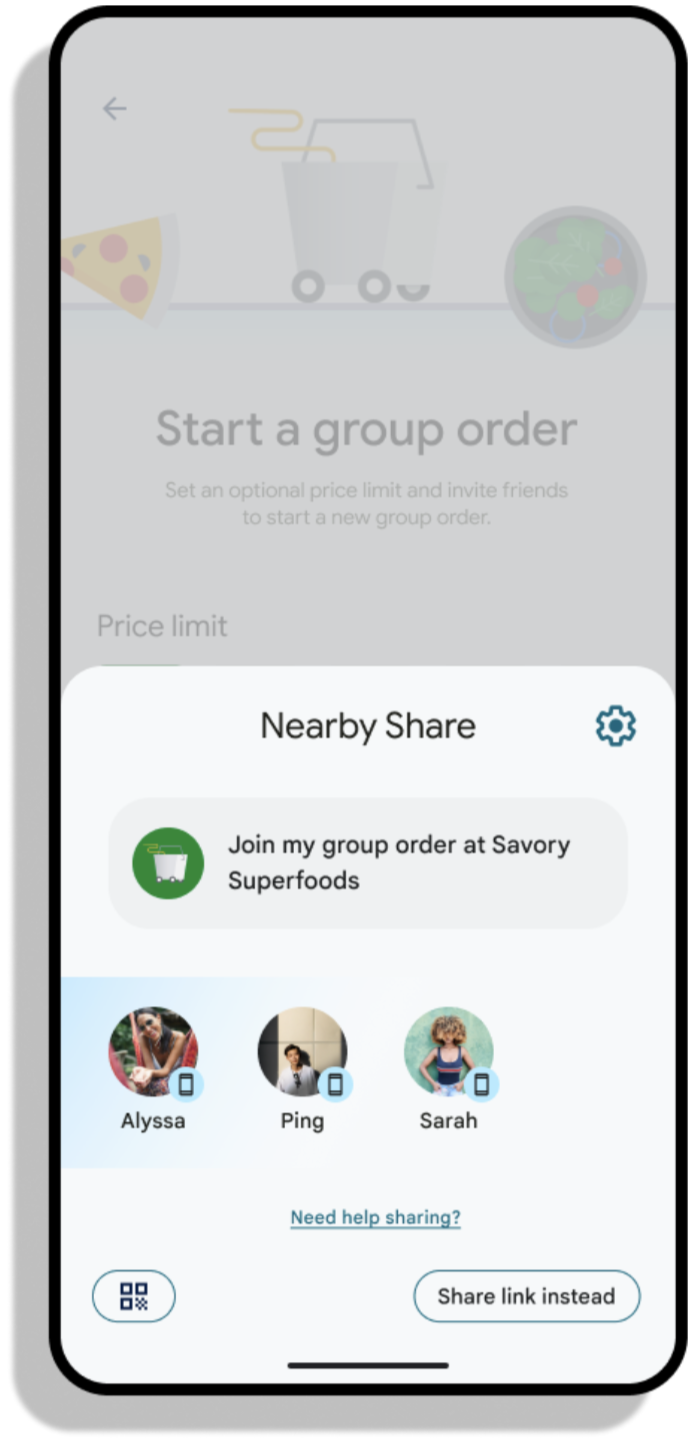
啟動裝置選取對話方塊
裝置探索功能會使用系統對話方塊,讓使用者選取目標裝置。目的地:
要啟動裝置選取對話方塊,您必須先取得裝置探索功能
並註冊結果接收器。請注意,與
registerForActivityResult
,這個接收器必須無條件註冊為
部分活動或片段初始化路徑。
Kotlin
override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) devicePickerLauncher = Discovery.create(this).registerForResult(this, handleDevices) }
Java
@Override public void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); devicePickerLauncher = Discovery.create(this).registerForResult(this, handleDevices); }
在上方的程式碼片段中,我們有未定義的 handleDevices
物件。更新後
使用者選擇要連結的裝置,以及 SDK 成功後要連線的裝置
這個回呼會連線至其他裝置,這個回呼會接收 Participants
清單
已選取。
Kotlin
handleDevices = OnDevicePickerResultListener { participants -> participants.forEach { // Use participant info } }
Java
handleDevices = participants -> { for (Participant participant : participants) { // Use participant info } }
註冊裝置選擇器後,使用 devicePickerLauncher
啟動裝置選擇器
執行個體。DevicePickerLauncher.launchDevicePicker
會使用兩個參數:
裝置篩選器清單 (請見下方章節) 和 startComponentRequest
。
startComponentRequest
用於指定要在哪個活動啟動的活動
以及使用者看到要求的原因。
Kotlin
devicePickerLauncher.launchDevicePicker( listOf(), startComponentRequest { action = "com.example.crossdevice.MAIN" reason = "I want to say hello to you" }, )
Java
devicePickerLauncher.launchDevicePickerFuture( Collections.emptyList(), new StartComponentRequest.Builder() .setAction("com.example.crossdevice.MAIN") .setReason("I want to say hello to you") .build());
接受連線要求
使用者在裝置挑選器中選取裝置後,「
接收裝置,要求使用者接受連線。接受之後,
目標活動啟動後,您可以在 onCreate
和
onNewIntent
。
Kotlin
override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) handleIntent(getIntent()) } override fun onNewIntent(intent: Intent) { super.onNewIntent(intent) handleIntent(intent) } private fun handleIntent(intent: Intent) { val participant = Discovery.create(this).getParticipantFromIntent(intent) // Accept connection from participant (see below) }
Java
@Override public void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); handleIntent(getIntent()); } @Override public void onNewIntent(Intent intent) { super.onNewIntent(intent); handleIntent(intent); } private void handleIntent(Intent intent) { Participant participant = Discovery.create(this).getParticipantFromIntent(intent); // Accept connection from participant (see below) }
裝置篩選器
找到裝置時,我們通常會選擇只篩選這些裝置 並展示與目前用途相關的字詞例如:
- 篩選範圍僅限配備攝影機的裝置,以便掃描 QR code
- 僅透過電視篩選,享受大螢幕觀影體驗
在這個開發人員預覽版中,我們將新增篩選功能,方便您 同一使用者擁有的裝置。
您可以使用 DeviceFilter
類別指定裝置篩選器:
Kotlin
val deviceFilters = listOf(DeviceFilter.trustRelationshipFilter(MY_DEVICES_ONLY))
Java
List<DeviceFilter> deviceFilters = Arrays.asList(DeviceFilter.trustRelationshipFilter(MY_DEVICES_ONLY));
定義裝置篩選器後,即可開始探索裝置。
Kotlin
devicePickerLauncher.launchDevicePicker(deviceFilters, startComponentRequest)
Java
Futures.addCallback( devicePickerLauncher.launchDevicePickerFuture(deviceFilters, startComponentRequest), new FutureCallback<Void>() { @Override public void onSuccess(Void result) { // do nothing, result will be returned to handleDevices callback } @Override public void onFailure(Throwable t) { // handle error } }, mainExecutor);
請注意,launchDevicePicker
是非同步函式,會使用
suspend
關鍵字。