QuoteSpan
public
class
QuoteSpan
extends Object
implements
LeadingMarginSpan,
ParcelableSpan
java.lang.Object | |
↳ | android.text.style.QuoteSpan |
A span which styles paragraphs by adding a vertical stripe at the beginning of the text (respecting layout direction).
A QuoteSpan
must be attached from the first character to the last character of a
single paragraph, otherwise the span will not be displayed.
QuoteSpans
allow configuring the following elements:
- color - the vertical stripe color. By default, the stripe color is 0xff0000ff
- gap width - the distance, in pixels, between the stripe and the paragraph. Default value is 2px.
- stripe width - the width, in pixels, of the stripe. Default value is 2px.
QuoteSpan
using the default values can be constructed like this:
SpannableString string = new SpannableString("Text with quote span on a long line");
string.setSpan(new QuoteSpan(), 0, string.length(), Spanned.SPAN_EXCLUSIVE_EXCLUSIVE);
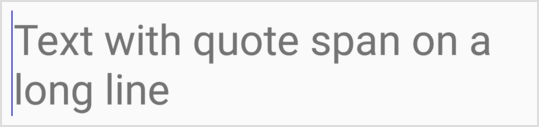
QuoteSpan
constructed with default values.
To construct a QuoteSpan
with a green stripe, of 20px in width and a gap width of
40px:
SpannableString string = new SpannableString("Text with quote span on a long line");
string.setSpan(new QuoteSpan(Color.GREEN, 20, 40), 0, string.length(),
Spanned.SPAN_EXCLUSIVE_EXCLUSIVE);
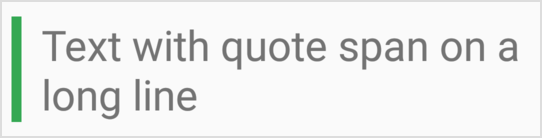
QuoteSpan
.Summary
Constants | |
---|---|
int |
STANDARD_COLOR
Default color for the quote stripe. |
int |
STANDARD_GAP_WIDTH_PX
Default gap width in pixels. |
int |
STANDARD_STRIPE_WIDTH_PX
Default stripe width in pixels. |
Inherited constants |
---|
Public constructors | |
---|---|
QuoteSpan()
Creates a |
|
QuoteSpan(Parcel src)
Create a |
|
QuoteSpan(int color)
Creates a |
|
QuoteSpan(int color, int stripeWidth, int gapWidth)
Creates a |
Public methods | |
---|---|
int
|
describeContents()
Describe the kinds of special objects contained in this Parcelable instance's marshaled representation. |
void
|
drawLeadingMargin(Canvas c, Paint p, int x, int dir, int top, int baseline, int bottom, CharSequence text, int start, int end, boolean first, Layout layout)
Renders the leading margin. |
int
|
getColor()
Get the color of the quote stripe. |
int
|
getGapWidth()
Get the width of the gap between the stripe and the text. |
int
|
getLeadingMargin(boolean first)
Returns the amount by which to adjust the leading margin. |
int
|
getSpanTypeId()
Return a special type identifier for this span class. |
int
|
getStripeWidth()
Get the width of the quote stripe. |
String
|
toString()
Returns a string representation of the object. |
void
|
writeToParcel(Parcel dest, int flags)
Flatten this object in to a Parcel. |
Inherited methods | |
---|---|
Constants
STANDARD_COLOR
public static final int STANDARD_COLOR
Default color for the quote stripe.
Constant Value: -16776961 (0xff0000ff)
STANDARD_GAP_WIDTH_PX
public static final int STANDARD_GAP_WIDTH_PX
Default gap width in pixels.
Constant Value: 2 (0x00000002)
STANDARD_STRIPE_WIDTH_PX
public static final int STANDARD_STRIPE_WIDTH_PX
Default stripe width in pixels.
Constant Value: 2 (0x00000002)
Public constructors
QuoteSpan
public QuoteSpan (Parcel src)
Create a QuoteSpan
from a parcel.
Parameters | |
---|---|
src |
Parcel : This value cannot be null . |
QuoteSpan
public QuoteSpan (int color)
Creates a QuoteSpan
based on a color.
Parameters | |
---|---|
color |
int : the color of the quote stripe. |
QuoteSpan
public QuoteSpan (int color, int stripeWidth, int gapWidth)
Creates a QuoteSpan
based on a color, a stripe width and the width of the gap
between the stripe and the text.
Parameters | |
---|---|
color |
int : the color of the quote stripe. |
stripeWidth |
int : the width of the stripe.
Value is 0 or greater |
gapWidth |
int : the width of the gap between the stripe and the text.
Value is 0 or greater |
Public methods
describeContents
public int describeContents ()
Describe the kinds of special objects contained in this Parcelable
instance's marshaled representation. For example, if the object will
include a file descriptor in the output of writeToParcel(android.os.Parcel, int)
,
the return value of this method must include the
CONTENTS_FILE_DESCRIPTOR
bit.
Returns | |
---|---|
int |
a bitmask indicating the set of special object types marshaled
by this Parcelable object instance.
Value is either 0 or CONTENTS_FILE_DESCRIPTOR |
drawLeadingMargin
public void drawLeadingMargin (Canvas c, Paint p, int x, int dir, int top, int baseline, int bottom, CharSequence text, int start, int end, boolean first, Layout layout)
Renders the leading margin. This is called before the margin has been
adjusted by the value returned by getLeadingMargin(boolean)
.
Parameters | |
---|---|
c |
Canvas : This value cannot be null . |
p |
Paint : This value cannot be null . |
x |
int : the current position of the margin |
dir |
int : the base direction of the paragraph; if negative, the margin
is to the right of the text, otherwise it is to the left. |
top |
int : the top of the line |
baseline |
int : the baseline of the line |
bottom |
int : the bottom of the line |
text |
CharSequence : This value cannot be null . |
start |
int : the start of the line |
end |
int : the end of the line |
first |
boolean : true if this is the first line of its paragraph |
layout |
Layout : This value cannot be null . |
getColor
public int getColor ()
Get the color of the quote stripe.
Returns | |
---|---|
int |
the color of the quote stripe. |
getGapWidth
public int getGapWidth ()
Get the width of the gap between the stripe and the text.
Returns | |
---|---|
int |
the width of the gap between the stripe and the text. |
getLeadingMargin
public int getLeadingMargin (boolean first)
Returns the amount by which to adjust the leading margin. Positive values move away from the leading edge of the paragraph, negative values move towards it.
Parameters | |
---|---|
first |
boolean : true if the request is for the first line of a paragraph,
false for subsequent lines |
Returns | |
---|---|
int |
the offset for the margin. |
getSpanTypeId
public int getSpanTypeId ()
Return a special type identifier for this span class.
Returns | |
---|---|
int |
getStripeWidth
public int getStripeWidth ()
Get the width of the quote stripe.
Returns | |
---|---|
int |
the width of the quote stripe. |
toString
public String toString ()
Returns a string representation of the object.
Returns | |
---|---|
String |
a string representation of the object. |
writeToParcel
public void writeToParcel (Parcel dest, int flags)
Flatten this object in to a Parcel.
Parameters | |
---|---|
dest |
Parcel : The Parcel in which the object should be written.
This value cannot be null . |
flags |
int : Additional flags about how the object should be written.
May be 0 or Parcelable.PARCELABLE_WRITE_RETURN_VALUE .
Value is either 0 or a combination of Parcelable.PARCELABLE_WRITE_RETURN_VALUE , and android.os.Parcelable.PARCELABLE_ELIDE_DUPLICATES |